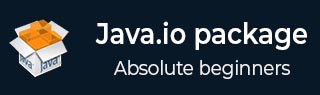
- Java.io 包类
- Java.io - 首页
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io 包额外内容
- Java.io - 接口
- Java.io - 异常
- Java.io 包有用资源
- Java.io - 讨论
Java - File canRead() 方法
描述
Java File canRead() 方法如果文件可以通过其抽象名称读取,则返回 true。
声明
以下是 java.io.File.canRead() 方法的声明:
public boolean canRead()
参数
无
返回值
此方法返回布尔值。如果路径名存在且应用程序允许读取文件,则返回 true。
异常
SecurityException - 如果 SecurityManager.checkRead(java.lang.String) 方法拒绝应用程序的读取访问权限。
示例 1
以下示例显示了 Java File canRead() 方法的用法。我们创建了一个 File 引用。然后,我们使用给定位置存在的文件创建一个 File 对象。使用 canRead() 方法,我们获取可读文件的可读状态。然后使用 getAbsolutePath(),我们获取文件的绝对路径。最后,我们打印文件名及其可读状态。
import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new file f = new File("F://test.txt"); // true if the file is readable boolean bool = f.canRead(); // find the absolute path String path = f.getAbsolutePath(); // prints System.out.println(path + " is readable: "+ bool); } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果 - 假设我们在当前位置有一个 test.txt 文件,并且不可读。
F:\test.txt is readable: true
示例 2
以下示例显示了 Java File canRead() 方法的用法。我们创建了一个 File 引用。然后,我们使用不可读的文件创建一个 File 对象。使用 canRead() 方法,我们获取文件的可读状态。然后使用 getAbsolutePath(),我们获取文件的绝对路径。最后,我们打印文件名及其可读状态。
import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new file f = new File("F://test1.txt"); // true if the file is readable boolean bool = f.canRead(); // find the absolute path String path = f.getAbsolutePath(); // prints System.out.println(path + " is readable: "+ bool); } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果 - 假设我们在给定位置有一个不可读的 test2.txt 文件。
F:\test1.txt is readable: false
示例 3
以下示例显示了 Java File canRead() 方法的用法。我们创建了一个 File 引用。然后,我们使用给定位置不存在的文件创建一个 File 对象。使用 canRead() 方法,我们获取文件的可读状态。然后使用 getAbsolutePath(),我们获取文件的绝对路径。最后,我们打印文件名及其可读状态。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new file f = new File("F://test2.txt"); // true if the file is readable boolean bool = f.canRead(); // find the absolute path String path = f.getAbsolutePath(); // prints System.out.println(path + " is readable: "+ bool); } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果 - 假设我们在给定位置没有 test2.txt 文件,因此不可读。
F:\test2.txt is readable: false