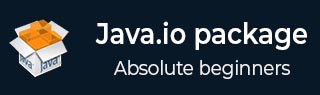
- Java.io 包类
- Java.io - 首页
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- Java.io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io 包额外内容
- Java.io - 接口
- Java.io - 异常
- Java.io 包实用资源
- Java.io - 讨论
Java - File exists() 方法
描述
Java File exists() 方法测试由该抽象路径名定义的文件或目录是否存在。
声明
以下是 java.io.File.exists() 方法的声明:
public boolean exists()
参数
无
返回值
当且仅当由抽象路径名定义的文件存在时,该方法返回布尔值 true;否则返回 false。
异常
无
示例 1
以下示例演示了 Java File exists() 方法的用法。我们创建了一个 File 引用。然后,我们使用当前目录中不存在的 test.txt 创建一个 File 对象。然后,我们使用 createNewFile() 方法创建文件。现在,使用 exists() 方法,我们检查文件是否已创建。然后,如果文件存在,我们将删除该文件,并在再次使用 exists() 方法检查后打印状态。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("test.txt"); // create new file in the system f.createNewFile(); // tests if file exists bool = f.exists(); // prints System.out.println("File exists: "+bool); if(bool == true) { // delete() invoked f.delete(); System.out.println("delete() invoked"); } // tests if file exists bool = f.exists(); // prints System.out.print("File exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
File exists: true delete() invoked File exists: false
示例 2
以下示例演示了 Java File exists() 方法的用法。我们创建了一个 File 引用。然后,我们使用提供的目录中存在的 F:/test.txt 创建一个 File 对象。现在,使用 exists() 方法,我们检查文件是否存在。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("F:/test.txt"); // tests if file exists bool = f.exists(); // prints System.out.println("File exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
File exists: true
示例 3
以下示例演示了 Java File exists() 方法的用法。我们创建了一个 File 引用。然后,我们使用提供的目录中存在的 F:/test 目录创建一个 File 对象。现在,使用 exists() 方法,我们检查目录是否存在。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("F:/test"); // tests if file exists bool = f.exists(); // prints System.out.println("Directory exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Directory exists: true
java_file_class.htm
广告