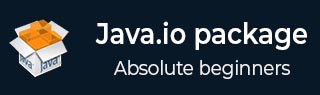
- Java.io 包类
- Java.io - 首页
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io 包扩展
- Java.io - 接口
- Java.io - 异常
- Java.io 包有用资源
- Java.io - 讨论
Java - File getParent() 方法
描述
Java File getParent() 方法返回此抽象路径名的父路径名字符串,如果此路径名未命名父目录,则返回 null。
声明
以下是 java.io.File.getParent() 方法的声明:-
public String getParent()
参数
无
返回值
此方法返回此抽象路径名命名的父目录的路径名字符串,如果路径名未命名父目录,则返回 null。
异常
无
示例 1
以下示例演示了 Java File getParent() 方法的使用。我们创建了两个 File 引用。然后我们使用当前目录中不存在的 test.txt 创建一个 File 对象。然后我们使用 createNewFile() 方法创建了该文件。现在使用 getAbsoluteFile() 方法获取文件,并使用 getParent() 方法获取文件的父目录名称并打印它。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; File f1 = null; String path = ""; boolean bool = false; try { // create new files f = new File("test.txt"); // create new file in the system f.createNewFile(); // create new file object from the absolute path f1 = f.getAbsoluteFile(); // returns true if the file exists bool = f1.exists(); // returns name of parent of the file path = f1.getParent(); // if file exists if(bool) { // prints the file System.out.print("Parent: " + path); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:-
Parent: F:\Workspace\Tester
示例 2
以下示例演示了 Java File getParent() 方法的使用。我们创建了一个 File 引用。然后我们使用提供的目录中的 F:/test.txt 创建一个 File 对象。现在使用 getAbsoluteFile() 方法获取文件,并在使用 getParent() 方法获取其名称后打印其父目录名称。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new files f = new File("F:/test.txt"); // get the file File f1 = f.getAbsoluteFile(); // prints the parent of file System.out.println("Parent: "+f1.getParent()); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:-
Parent: F:\
示例 3
以下示例演示了 Java File getParent() 方法的使用。我们创建了一个 File 引用。然后我们使用提供的路径中的 F:/test 目录创建一个 File 对象。现在使用 getAbsoluteFile() 方法获取目录,并使用 getParent() 方法获取其父目录名称。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new files f = new File("F:/test"); // get the file File f1 = f.getAbsoluteFile(); // prints the file parent name System.out.println("Parent Directory: "+f1.getParent()); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:-
Parent Directory: F:\
java_file_class.htm
广告