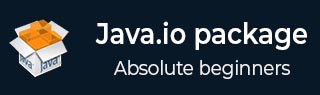
- Java.io 包类
- Java.io - 首页
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io 包额外内容
- Java.io - 接口
- Java.io - 异常
- Java.io 包有用资源
- Java.io - 讨论
Java - File list() 方法
描述
Java 的File list()方法返回由该抽象路径名定义的目录中文件和目录的数组。如果抽象路径名不表示目录,则该方法返回 null。
声明
以下是java.io.File.list()方法的声明:
public String[] list()
参数
无
返回值
该方法返回由该抽象路径名表示的目录中的文件和目录数组。
异常
SecurityException - 如果存在安全管理器并且其SecurityManager.checkRead(java.lang.String)方法拒绝读取文件的访问权限
示例 1
以下示例演示了 Java File list() 方法的用法。我们创建了一个 File 引用。然后我们使用提供的目录中存在的 test 目录创建一个 File 对象。然后我们使用 list() 方法将 test 目录中存在的文件列表获取到一个字符串数组中。然后迭代此数组以打印给定目录中可用的文件。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; String[] paths; try { // create new file f = new File("F:/test"); // array of files and directory paths = f.list(); // for each name in the path array for(String path:paths) { // prints filename and directory name System.out.println(path); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
test.txt Test1.txt
示例 2
以下示例演示了 Java File list() 方法的用法。我们创建了一个 File 引用。然后我们使用我们正在运行此程序的当前目录“.”创建一个 File 对象。然后我们使用 list() 方法将当前目录中存在的文件列表获取到一个字符串数组中。然后迭代此数组以打印给定目录中可用的文件。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; String[] paths; try { // create new file f = new File("."); // array of files and directory paths = f.list(); // for each name in the path array for(String path:paths) { // prints filename and directory name System.out.println(path); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
.classpath .project .settings app.log bin properties.txt properties.xml src test.txt
示例 3
以下示例演示了 Java File list() 方法的用法。我们创建了一个 File 引用。然后我们使用提供的目录中不存在的 test3 目录创建一个 File 对象。然后我们使用 list() 方法将 test 目录中存在的文件列表获取到一个字符串数组中。然后迭代此数组以打印给定目录中可用的文件。由于文件夹不存在,数组将为 null,我们打印一条消息 - “文件不存在”。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; String[] paths; try { // create new file f = new File("F:/Test3"); // array of files and directory paths = f.list(); if(paths != null) { // for each name in the path array for(String path:paths) { // prints filename and directory name System.out.println(path); } }else { System.out.println("Files are not present"); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Files are not present