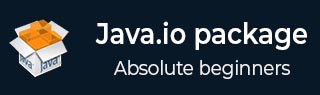
- Java.io 包类
- Java.io - 首页
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- Java.io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io 包附加内容
- Java.io - 接口
- Java.io - 异常
- Java.io 包有用资源
- Java.io - 讨论
Java - File toString() 方法
描述
Java File toString() 方法返回此抽象路径名的 getPath() 方法返回的路径名字符串。
声明
以下是 java.io.File.toString() 方法的声明:
1public String toString()
参数
无
返回值
该方法返回此抽象路径名的字符串形式。
异常
无
示例 1
以下示例演示了 Java File toString() 方法的用法。我们创建了两个 File 引用。然后,我们使用当前目录中不存在的 test.txt 创建一个 File 对象。然后,我们使用 createNewFile() 方法创建该文件。现在,使用 getAbsoluteFile() 方法获取文件,并使用 toString() 方法获取文件的字符串表示形式(即其路径),然后我们使用 exists() 方法检查文件是否存在。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; File f1 = null; String path = ""; boolean bool = false; try { // create new files f = new File("test.txt"); // create new file in the system f.createNewFile(); // create new file object from the absolute path f1 = f.getAbsoluteFile(); // returns true if the file exists bool = f1.exists(); // returns string representation of the file path = f1.toString(); // if file exists if(bool) { // prints the file System.out.print(path+" Exists? "+ bool); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
F:\Workspace\Tester\test.txt Exists? true
示例 2
以下示例演示了 Java File toString() 方法的用法。我们创建了一个 File 引用。然后,我们使用 F:/test.txt(存在于提供的目录中)创建了一个 File 对象。现在,使用 getAbsoluteFile() 方法获取文件,并使用 toString() 方法打印其字符串表示形式(即路径)。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new files f = new File("F:/Test2/test.txt"); // get the file File f1 = f.getAbsoluteFile(); // prints string representation of the file System.out.println("File: "+f1.toString()); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
File: F:\Test2\test.txt
示例 3
以下示例演示了 Java File toString() 方法的用法。我们创建了一个 File 引用。然后,我们使用 F:/test 目录(存在于提供的目录中)创建了一个 File 对象。现在,使用 getAbsoluteFile() 方法获取目录及其字符串表示形式(即路径)。
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; try { // create new files f = new File("F:/test2"); // get the file File f1 = f.getAbsoluteFile(); // prints the string representation of the file System.out.println("Directory: "+f1.toString()); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Directory: F:\test2
java_file_class.htm
广告