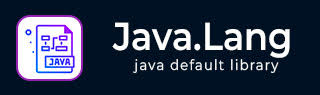
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Boolean compareTo() 方法
描述
Java Boolean compareTo(Boolean b) 方法用于比较此 Boolean 实例与另一个实例。
声明
以下是 java.lang.Boolean.compareTo() 方法的声明
public int compareTo(Boolean b)
指定于
接口 Comparable<Boolean> 中的 compareTo
参数
b − 要比较的 Boolean 实例
返回值
此方法返回:
零 − 如果此对象表示与参数相同的布尔值
正值 − 如果此对象表示 true 且参数表示 false
负值 − 如果此对象表示 false 且参数表示 true。
异常
NullPointerException − 如果参数为 null
比较 true 和 false 布尔值对象的示例
以下示例演示了如何使用 Boolean compareTo() 方法与 Boolean 对象。在此程序中,我们创建了两个 Boolean 变量,并分别为它们分配了值为 true 和 false 的 Boolean 对象。使用 compareTo() 方法比较这两个对象,并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // assign values to b1, b2 b1 = Boolean.valueOf(true); b2 = Boolean.valueOf(false); // create an int res int res; // compare b1 with b2 res = b1.compareTo(b2); String str1 = "Both values are equal "; String str2 = "Object value is true"; String str3 = "Argument value is true"; if( res == 0 ) { System.out.println( str1 ); } else if( res > 0 ) { System.out.println( str2 ); } else if( res < 0 ) { System.out.println( str3 ); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Object value is true
比较 true 和 false 布尔值基本类型的示例
以下示例演示了如何使用 Boolean compareTo() 方法与 Boolean 对象。在此程序中,我们创建了两个 Boolean 变量,并分别为它们分配了值为 true 和 false 的布尔值基本类型。使用 compareTo() 方法比较这两个对象,并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // assign values to b1, b2 b1 = true; b2 = false; // create an int res int res; // compare b1 with b2 res = b1.compareTo(b2); String str1 = "Both values are equal "; String str2 = "Object value is true"; String str3 = "Argument value is true"; if( res == 0 ) { System.out.println( str1 ); } else if( res > 0 ) { System.out.println( str2 ); } else if( res < 0 ) { System.out.println( str3 ); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Object value is true
比较 true 和 false 布尔值对象的示例
以下示例演示了如何使用 Boolean compareTo() 方法与 Boolean 对象。在此程序中,我们创建了两个 Boolean 变量,一个分配了值为 true 的布尔值基本类型,另一个分配了值为 false 的 Boolean 对象。使用 compareTo() 方法比较这两个对象,并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // assign values to b1, b2 b1 = true; b2 = Boolean.valueOf(false); // create an int res int res; // compare b1 with b2 res = b1.compareTo(b2); String str1 = "Both values are equal "; String str2 = "Object value is true"; String str3 = "Argument value is true"; if( res == 0 ) { System.out.println( str1 ); } else if( res > 0 ) { System.out.println( str2 ); } else if( res < 0 ) { System.out.println( str3 ); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Object value is true