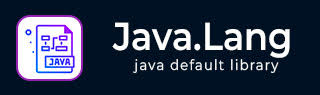
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Boolean equals() 方法
描述
Java Boolean equals(Object obj) 方法仅当参数不为 null 且是表示与此对象相同布尔值的 Boolean 对象时才返回 true。
声明
以下是java.lang.Boolean.equals() 方法的声明
public boolean equals(Object obj)
重写
Object 类中的 equals 方法
参数
obj − 要比较的对象
返回值
如果 Boolean 对象表示相同的值,则此方法返回 true;否则返回 false。
异常
无
使用 true 和 false 值检查 Boolean 对象相等性的示例
以下示例演示了使用 Boolean 对象的 Boolean equals() 方法。在此程序中,我们创建了两个 Boolean 变量,并为它们分配了具有 true 和 false 值的两个 Boolean 对象。使用 equals() 方法,比较两个对象的相等性并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // create a boolean primitive res boolean res; // assign values to b1, b2 b1 = Boolean.valueOf(true); b2 = Boolean.valueOf(false); // assign the result of equals method on b1, b2 to res res = b1.equals(b2); String str = "b1:" +b1+ " and b2:" +b2+ " are equal is " + res; // print res value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
b1:true and b2:false are equal is false
使用原始 true 和 false 值检查 Boolean 对象相等性的示例
以下示例演示了使用 Boolean 对象的 Boolean equals() 方法。在此程序中,我们创建了两个 Boolean 变量,并为它们分配了具有 true 和 false 原始值的两个 Boolean 对象。使用 equals() 方法,比较两个对象的相等性并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // create a boolean primitive res boolean res; // assign values to b1, b2 b1 = Boolean.valueOf(true); b2 = false; // assign the result of equals method on b1, b2 to res res = b1.equals(b2); String str = "b1:" +b1+ " and b2:" +b2+ " are equal is " + res; // print res value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
b1:true and b2:false are equal is false
以下示例演示了使用 Boolean 对象的 Boolean equals() 方法,其中包含 true 和 null 值。使用 equals() 方法,比较两个对象的相等性并打印结果。
以下是如何使用 null 值的另一个 Boolean equals() 方法示例。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // create a boolean primitive res boolean res; // assign values to b1, b2 b1 = Boolean.valueOf(true); b2 = null; // assign the result of equals method on b1, b2 to res res = b1.equals(b2); String str = "b1:" +b1+ " and b2:" +b2+ " are equal is " + res; // print res value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
b1:true and b2:null are equal is false
java_lang_boolean.htm
广告