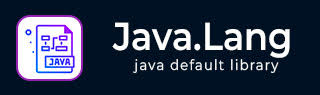
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Boolean getBoolean() 方法
描述
Java Boolean getBoolean(String name) 方法仅当由参数命名的系统属性存在且等于字符串“true”时才返回 true。系统属性可以通过 getProperty 方法访问,该方法由 System 类定义。
如果没有指定名称的属性,或者指定的名称为空或为 null,则返回 false。
声明
以下是 java.lang.Boolean.getBoolean() 方法的声明
public static boolean getBoolean(String name)
参数
name − 系统属性名称
返回值
此方法返回系统属性的布尔值。
异常
无
使用系统属性 True 获取布尔值示例
以下示例演示了 Boolean getBoolean() 方法的使用,其中系统属性存在且值为 true 和随机字符串“abcd”。在此程序中,我们创建了两个布尔变量。使用 System.setProperty(),我们创建了两个系统属性 demo1 和 demo2,其值分别为 true 和 abcd。现在使用 getBoolean() 方法,检索系统属性的值并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 boolean primitives bool1, bool2 boolean bool1, bool2; /** * using System class's setProprty method, set the values of * system properties demo1, demo2. */ System.setProperty("demo1","true"); System.setProperty("demo2","abcd"); // retrieve value of system properties to s1, s2 String s1 = System.getProperty("demo1"); String s2 = System.getProperty("demo2"); // assign result of getBoolean on demo1, demo2 to bool1, bool2 bool1 = Boolean.getBoolean("demo1"); bool2 = Boolean.getBoolean("demo2"); String str1 = "boolean value of system property demo1 is " + bool1; String str2 = "System property value of demo1 is " + s1; String str3 = "boolean value of system property demo2 is " + bool2; String str4 = "System property value of demo2 is " + s2; // print bool1, bool2 and s1, s2 values System.out.println( str1 ); System.out.println( str2 ); System.out.println( str3 ); System.out.println( str4 ); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
boolean value of system property demo1 is true System property value of demo1 is true boolean value of system property demo2 is false System property value of demo2 is abcd
使用系统属性获取布尔值示例
以下示例演示了 Boolean getBoolean() 方法的使用,其中系统属性存在且值为 false 和随机字符串“abcd”。在此程序中,我们创建了两个布尔变量。使用 System.setProperty(),我们创建了两个系统属性 demo1 和 demo2,其值分别为 false 和 abcd。现在使用 getBoolean() 方法,检索系统属性的值并打印结果。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 boolean primitives bool1, bool2 boolean bool1, bool2; /** * using System class's setProprty method, set the values of * system properties demo1, demo2. */ System.setProperty("demo1","false"); System.setProperty("demo2","abcd"); // retrieve value of system properties to s1, s2 String s1 = System.getProperty("demo1"); String s2 = System.getProperty("demo2"); // assign result of getBoolean on demo1, demo2 to bool1, bool2 bool1 = Boolean.getBoolean("demo1"); bool2 = Boolean.getBoolean("demo2"); String str1 = "boolean value of system property demo1 is " + bool1; String str2 = "System property value of demo1 is " + s1; String str3 = "boolean value of system property demo2 is " + bool2; String str4 = "System property value of demo2 is " + s2; // print bool1, bool2 and s1, s2 values System.out.println( str1 ); System.out.println( str2 ); System.out.println( str3 ); System.out.println( str4 ); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
boolean value of system property demo1 is false System property value of demo1 is false boolean value of system property demo2 is false System property value of demo2 is abcd
使用不存在的系统属性获取布尔值示例
以下示例演示了 Boolean getBoolean() 方法的使用,其中系统属性不存在。在此程序中,我们创建了两个布尔变量。现在使用 getBoolean() 方法,检索系统属性的值并打印结果。由于系统属性不存在,因此 getBoolean 方法返回 false。
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 boolean primitives bool1, bool2 boolean bool1, bool2; // retrieve value of system properties to s1, s2 String s1 = System.getProperty("demo1"); String s2 = System.getProperty("demo2"); // assign result of getBoolean on demo1, demo2 to bool1, bool2 bool1 = Boolean.getBoolean("demo1"); bool2 = Boolean.getBoolean("demo2"); String str1 = "boolean value of system property demo1 is " + bool1; String str2 = "System property value of demo1 is " + s1; String str3 = "boolean value of system property demo2 is " + bool2; String str4 = "System property value of demo2 is " + s2; // print bool1, bool2 and s1, s2 values System.out.println( str1 ); System.out.println( str2 ); System.out.println( str3 ); System.out.println( str4 ); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
boolean value of system property demo1 is false System property value of demo1 is null boolean value of system property demo2 is false System property value of demo2 is null