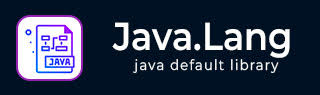
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang -有用资源
- Java.lang - 讨论
Java - Byte valueOf() 方法
描述
Java Byte valueOf(String s, int radix) 方法返回一个 Byte 对象,该对象保存从指定字符串中提取的值,该值使用第二个参数给出的基数进行解析。
第一个参数被解释为代表一个用第二个参数指定的基数表示的有符号字节,就像该参数被提供给 parseByte(java.lang.String, int) 方法一样。结果是一个 Byte 对象,它表示字符串指定的字节值。
声明
以下是 java.lang.Byte.valueOf() 方法的声明
public static Byte valueOf(String s, int radix)throws NumberFormatException
参数
s − 要解析的字符串
radix − 用于解释 s 的基数
返回值
此方法返回一个 Byte 对象,该对象保存由字符串参数在指定基数中表示的值。
异常
NumberFormatException − 如果字符串不包含可解析的字节。
使用基数 2 解析包含减号的字符串以获取字节示例
以下示例显示了 Byte valueOf(String,int) 方法的用法。我们创建了一个字符串变量并为其赋值。然后创建一个 Byte 变量,并使用 Byte.valueOf(String,, int) 方法,使用基数 2 解析字符串的值并打印。
package com.tutorialspoint; public class ByteDemo { public static void main(String[] args) { // create a String s and assign value to it String s = "-1010"; // create a Byte object b Byte b; /** * static method is called using class name. * assign Byte instance value of s to b using radix as 2 * radix 2 represents binary */ b = Byte.valueOf(s, 2); String str = "Byte value of string " + s + " using radix 2 is " + b; // print b value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Byte value of string -1010 using radix 2 is -10
使用基数 8 解析包含减号的字符串以获取字节示例
以下示例显示了 Byte valueOf(String,int) 方法的用法。我们创建了一个字符串变量并为其赋值。然后创建一个 Byte 变量,并使用 Byte.valueOf(String,, int) 方法,使用基数 8 解析字符串的值并打印。
package com.tutorialspoint; public class ByteDemo { public static void main(String[] args) { // create a String s and assign value to it String s = "-101"; // create a Byte object b Byte b; /** * static method is called using class name. * assign Byte instance value of s to b using radix as 8 * radix 8 represents octal */ b = Byte.valueOf(s, 8); String str = "Byte value of string " + s + " using radix 8 is " + b; // print b value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Byte value of string -101 using radix 8 is -65
使用基数 10 解析包含减号的字符串以获取字节示例
以下示例显示了 Byte valueOf(String,int) 方法的用法。我们创建了一个字符串变量并为其赋值。然后创建一个 Byte 变量,并使用 Byte.valueOf(String,, int) 方法,使用基数 10 解析字符串的值并打印。
package com.tutorialspoint; public class ByteDemo { public static void main(String[] args) { // create a String s and assign value to it String s = "-101"; // create a Byte object b Byte b; /** * static method is called using class name. * assign Byte instance value of s to b using radix as 10 * radix 8 represents decimal */ b = Byte.valueOf(s, 10); String str = "Byte value of string " + s + " using radix 10 is " + b; // print b value System.out.println( str ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Byte value of string -101 using radix 10 is -101