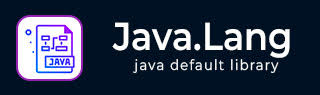
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Character codePointAt() 方法
描述
Java 的Character codePointAt()方法将检索字符数组中指定索引处的字符的代码点。这些代码点可以表示普通字符和补充字符。
当字符数组中特定索引处的值满足以下条件时,将获取补充代码点字符:
如果字符值属于高代理范围 (U+D800 到 U+DBFF)。
如果给定索引的下一个索引小于字符数组的长度。
如果此下一个索引中的值属于低代理范围 (U+DC00 到 U+DFFF)。
在任何其他情况下,将获取普通代码点字符。
注意 - 此方法以三种不同的多态形式出现,并具有不同的参数。
语法
以下是 Java Character codePointAt() 方法的各种语法
public static int codePointAt(char[] a, int index) (or) public static int codePointAt(char[] a, int index, int limit) (or) public static int codePointAt(CharSequence seq, int index)
参数
a - 字符数组
index - 要转换的字符数组中字符值(Unicode 代码单元)的索引
limit - 字符数组中最后一个可使用的数组元素之后的索引。
seq - 一系列字符值(Unicode 代码单元)
返回值
此方法返回字符数组/CharSequence 参数给定索引处的 Unicode 代码点。
获取字符数组中给定索引处的字符的代码点示例
以下示例演示了 Java Character codePointAt() 方法的使用。当我们将一个简单的字符数组和一个指定的小于数组长度的索引作为方法的参数时,返回值将是该索引处存在的字符的代码点。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create 2 int's res, index and assign value to index int res, index = 0; // assign result of codePointAt on array c at index to res res = Character.codePointAt(c, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
让我们编译并运行上述程序,这将产生以下结果:
Unicode code point is 97
获取字符数组中每个字符的代码点示例
在下面的另一个示例中,我们使用循环语句来检索数组中每个元素的代码点。通过使用循环语句将每个索引作为参数传递给方法,可以检索给定字符数组中每个元素的代码点。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c_arr = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create 2 int's res, index and assign value to index int res, index; // using a for loop assign the codePointAt on array c at index to res for (index = 0; index < c_arr.length; index++){ res = Character.codePointAt(c_arr, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } } }
如果您编译上面的代码,则输出将获得如下:
Unicode code point is 97 Unicode code point is 98 Unicode code point is 99 Unicode code point is 100 Unicode code point is 101
使用限制获取字符数组中给定索引处的字符的代码点示例
我们可以通过传递额外的参数“limit”来限制此字符数组中的搜索。在这种情况下,该方法将仅执行到给定限制的搜索并返回代码点。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] { 'a', 'b', 'c', 'd', 'e' }; // create and assign value to integers index, limit int index = 1, limit = 3; // create an int res int res; // assign result of codePointAt on array c at index to res using limit res = Character.codePointAt(c, index, limit); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
让我们编译并运行上述程序,这将产生以下结果:
Unicode code point is 98
使用无效限制在获取字符数组中给定索引处的字符的代码点时遇到异常示例
但是,如果 index 参数大于 array 参数的长度或 limit 参数,则该方法会抛出 IndexOutOfBounds 异常。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a char array c and assign values char[] c = new char[] {'a', 'b', 'c', 'd', 'e'}; // assign an index value greater than array length int res, index = 6; // assign result of codePointAt on array c at index to res res = Character.codePointAt(c, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
异常
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 6at java.lang.Character.codePointAtImpl(Character.java:4952) at java.lang.Character.codePointAt(Character.java:4915) at com.tutorialspoint.CharacterDemo.main(CharacterDemo.java:16)
获取CharSequence中给定索引处的字符的代码点示例
当我们将 CharSequence 和索引值作为参数传递给 codePointAt(CharSequence seq, int index) 方法时,返回值是给定 CharSequence 中索引处存在的字符。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a CharSequence seq and assign value CharSequence seq = "Hello"; // create and assign value to index int index = 4; // create an int res int res; // assign result of codePointAt on seq at index to res res = Character.codePointAt(seq, index); String str = "Unicode code point is " + res; // print res value System.out.println( str ); } }
让我们编译并运行上述程序,这将产生以下结果:
Unicode code point is 111
在获取 null CharSequence 中给定索引处的字符的代码点时遇到异常示例
但是,如果 CharSequence 初始化为 null 值并作为参数传递给方法,则会引发 NullPointer 异常。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a CharSequence seq and assign value CharSequence seq = null; // create and assign value to index int index = 5; // create an int res int res; // assign result of codePointAt on seq at index to res res = Character.codePointAt(seq, index); // print res value System.out.println("Unicode code point is " + res); } }
异常
Exception in thread "main" java.lang.NullPointerExceptionat java.lang.Character.codePointAt(Character.java:4883)