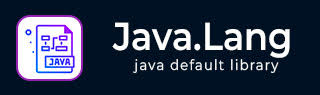
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - Character forDigit() 方法
Java 的 Character forDigit() 方法用于确定指定基数中特定数字的字符表示。
基数定义为数字系统中唯一数字(包括零)的数量。最常见的基数是 10,表示十进制系统 (0-9)。
使用此方法的优势在于,它允许您生成并非真正“数字”的数字。例如,在十六进制数字系统中,'9' 之后的数字使用字符 'A' 到 'F' 表示。
语法
以下是 Java Character forDigit() 方法的语法
public static char forDigit(int digit, int radix)
参数
digit − 要转换为字符的数字。如果 0 ≤ digit < radix,则此参数有效。
radix − 基数。如果 MIN_RADIX ≤ radix ≤ MAX_RADIX,则此参数有效。
返回值
此方法返回指定基数中指定数字的 char 表示。一些特殊情况包括:
如果 radix 的值不是有效的基数,或者 digit 的值不是指定基数中有效的数字,则返回空字符 ('\u0000')。
如果 digit 小于 10,则返回 '0' + digit。否则,返回 'a' + digit − 10。
获取特定数字和给定基数的字符表示示例
以下示例显示了 Java Character forDigit() 方法的使用方法。我们创建了两个 int 变量并用 int 值初始化,然后使用 forDigit() 方法,我们检索了 10 和 16 基数的 char 表示,并打印了结果。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create 2 character primitives ch1, ch2 char ch1, ch2; // create 2 int primitives i1, i2 and assign values int i1 = 3; int i2 = 14; // assign char representation of i1, i2 to ch1, ch2 using radix ch1 = Character.forDigit(i1, 10); ch2 = Character.forDigit(i2, 16); String str1 = "Char representation of " + i1 + " in radix 10 is " + ch1; String str2 = "Char representation of " + i2 + " in radix 16 is " + ch2; // print ch1, ch2 values System.out.println( str1 ); System.out.println( str2 ); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Char representation of 3 in radix 10 is 3 Char representation of 14 in radix 16 is e
获取超出数字和给定基数的字符表示示例
在此示例中,如果传递给方法的参数超过 MAX_RADIX,则返回值将为空字符。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create 2 character primitives ch1, ch2 char ch1, ch2; // create 2 int primitives i1, i2 and assign values int i1 = 15; int i2 = 20; // assign char representation of i1, i2 to ch1, ch2 using radix ch1 = Character.forDigit(i1, 10); ch2 = Character.forDigit(i2, 16); // print ch1, ch2 values System.out.println("Char representation of " + i1 + " in radix 10 is " + ch1); System.out.println("Char representation of " + i2 + " in radix 16 is " + ch2); } }
输出
编译上述代码后,获得的输出为:
Char representation of 100 in radix 10 is Char representation of 150 in radix 16 is
获取超出数字和给定基数的字符表示示例
负数不属于任何数字系统的类别,因为它在 MIN_RADIX 之前。因此,该方法返回空字符。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { char ch1, ch2; int i1 = -4; int i2 = -1; ch1 = Character.forDigit(i1, 10); ch2 = Character.forDigit(i2, 16); System.out.println("Char representation of " + i1 + " in radix 10 is " + ch1); System.out.println("Char representation of " + i2 + " in radix 16 is " + ch2); } }
输出
执行上述代码后,获得的输出为:
Char representation of -4 in radix 10 is Char representation of -1 in radix 16 is
我们可以看到负整数没有输出。
获取由 ASCII 值表示的数字和给定基数的字符表示示例
即使 '9' 之后的数字由字符 'a' 到 'f' 表示,这些字符的 ASCII 值也超出基数限制。因此,返回空字符。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { char ch1, ch2; int i1 = (int)'a'; int i2 = (int)'f'; ch1 = Character.forDigit(i1, 16); ch2 = Character.forDigit(i2, 16); System.out.println("Char representation of " + i1 + " in radix 16 is " + ch1); System.out.println("Char representation of " + i2 + " in radix 16 is " + ch2); } }
输出
获得的输出如下:
Char representation of 65 in radix 10 is . Char representation of 88 in radix 10 is .