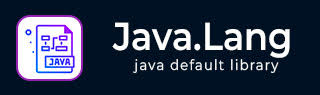
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - Character toChars() 方法
描述
Java 的 Character toChars() 方法将指定的字符(Unicode 代码点)转换为存储在 char 数组中的 UTF-16 表示形式。
Unicode 代码点分为两类:基本多语言平面 (BMP) 字符和补充字符。BMP 字符是已用 16 位 Unicode 表示形式表示的普通字符,而补充字符则不是。
如果指定的代码点是 BMP 值,则结果 char 数组的值与 codePoint 相同。如果指定的代码点是补充代码点,则结果 char 数组具有相应的代理对。
此方法以两种多态形式出现,具有不同的参数。
语法
以下是 Java Character toChars() 方法的语法
public static char[] toChars(int codePoint) (or) public static int toChars(int codePoint, char[] dst, int dstIndex)
参数
codePoint − Unicode 代码点
dst − char 数组,其中存储 codePoint 的 UTF-16 值
dstIndex − dst 数组中存储转换值的起始索引
返回值
此方法返回一个 char 数组,其中包含 codePoint 的 UTF-16 表示形式。
从代码点获取字符数组示例
以下示例显示了 Java Character toChars(int codePoint) 方法的使用方法。在此程序中,我们创建了一个 int 变量并将其初始化为一个代码点,并使用 toChars() 方法将结果 char 数组存储在一个数组中,并打印结果。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create a char array ch char ch[]; // create an int primitive cp and assign value int cp = 0x006e; // assign result of toChars on cp to ch ch = Character.toChars(cp); String str = "Char array having cp's UTF-16 representation is "; System.out.print( str ); // use a for loop to print ch for (int i = 0; i < ch.length; i++) { System.out.print( ch[i] ); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Char array having cp's UTF-16 representation is n
使用给定索引从字符获取代码点字符数组示例
以下示例显示了 Java Character toChars(int codePoint, char[] dst, int dstIndex) 方法的使用方法。在此程序中,我们创建了一个 int 变量并将其初始化为一个代码点,并使用 toChars() 方法将结果 char 数组存储在一个数组中,并打印结果。
package com.tutorialspoint; public class CharacterDemo { public static void main(String[] args) { // create an int primitive cp and assign value int cp = 0x0036; // create an int primitive res int res; /** * create a char array dst and assign UTF-16 value of cp * to it using toChars method */ char dst[] = Character.toChars(cp); // check if cp is BMP or supplementary code point using toChars res = Character.toChars(cp, dst, 0); String str1 = "It is a BMP code point"; String str2 = "It is a is a supplementary code point"; // print res value if ( res == 1 ) { System.out.println( str1 ); } else if ( res == 2 ) { System.out.println( str2 ); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
It is a BMP code point
获取代码点字符数组时遇到 IllegalArgumentException 示例
以下示例显示了 Java Character toChars(int codePoint) 方法的使用方法。在此程序中,我们创建了一个 int 变量并将其初始化为一个代码点,并使用 toChars() 方法将结果 char 数组存储在一个数组中,并打印结果。
下面给出的示例程序显示了方法抛出 IllegalArgumentException 的场景
public class Demo { public static void main(String[] args) { char ch[]; int cp = 0x2388211; ch = Character.toChars(cp); System.out.print("Char array having cp's UTF-16 representation is "); for (int i = 0; i < ch.length; i++) { System.out.println(ch[i]); } } }
异常
上面给出的程序首先被编译和运行,以产生以下输出结果。它抛出一个异常,因为给定方法的输入是非法的 Unicode 代码点。
Exception in thread "main" java.lang.IllegalArgumentException: Not a valid Unicode code point: 0x2388211 at java.base/java.lang.Character.toChars(Character.java:8573) at Demo.main(Demo.java:9)
获取代码点字符数组时遇到 NullPointerException 示例
以下示例显示了 Java Character toChars(int codePoint) 方法的使用方法。在此程序中,我们创建了一个 int 变量并将其初始化为一个代码点,并使用 toChars() 方法将结果 char 数组存储在一个数组中,并打印结果。
在这种情况下,方法抛出一个 NullPointerException。
public class Demo { public static void main(String[] args) { int cp = 0x1271; int res; res = Character.toChars(cp, null, 0); if ( res == 1 ) { System.out.println("It is a BMP code point"); } else if ( res == 2 ) { System.out.println("It is a is a supplementary code point"); } } }
异常
让我们编译并运行上面给出的程序,输出结果如下:抛出一个 NullPointerException:
Exception in thread "main" java.lang.NullPointerException at java.base/java.lang.Character.toChars(Character.java:8537) at Demo.main(Demo.java:11)