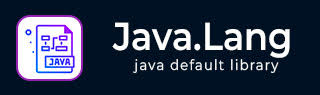
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java 类 getGenericInterfaces() 方法
描述
Java 类 getGenericInterfaces() 方法返回表示此对象所表示的类或接口直接实现的接口的类型。
声明
以下是 java.lang.Class.getGenericInterfaces() 方法的声明
public Type[] getGenericInterfaces()
参数
无
返回值
此方法返回此类实现的接口数组。
异常
GenericSignatureFormatError − 如果泛型类签名不符合 Java 虚拟机规范第 3 版中指定的格式。
TypeNotPresentException − 如果任何泛型超接口引用不存在的类型声明
MalformedParameterizedTypeException − 如果任何泛型超接口引用无法出于任何原因实例化的参数化类型。
没有接口的类的泛型接口示例
以下示例显示了 java.lang.Class.getGenericInterfaces() 方法的使用。在此程序中,我们创建了 ClassDemo 的实例,然后使用 getClass() 方法检索实例的类。使用 getGenericInterfaces(),我们检索了所有接口(如果已实现),否则打印备用消息。
package com.tutorialspoint; import java.lang.reflect.Type; public class ClassDemo { public static void main(String []args) { ClassDemo d = new ClassDemo(); Class c = d.getClass(); Type[] t = c.getGenericInterfaces(); if(t.length != 0) { for(Type val : t) { System.out.println(val.toString()); } } else { System.out.println("Interfaces are not implemented..."); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Interfaces are not implemented...
实现接口的类的泛型接口示例
以下示例显示了 java.lang.Class.getGenericInterfaces() 方法的使用。在此程序中,我们创建了 ClassDemo 的实例,然后使用 getClass() 方法检索实例的类。使用 getGenericInterfaces(),我们检索了所有接口(如果已实现),否则打印备用消息。
package com.tutorialspoint; import java.lang.reflect.Type; interface Print { public void show(); } public class ClassDemo implements Print { public void show(){ System.out.println("Class Demo"); } public static void main(String []args) { ClassDemo d = new ClassDemo(); Class c = d.getClass(); Type[] t = c.getGenericInterfaces(); if(t.length != 0) { for(Type val : t) { System.out.println(val.toString()); } } else { System.out.println("Interfaces are not implemented..."); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
interface com.tutorialspoint.Print
ArrayList 的泛型接口示例
以下示例显示了 java.lang.Class.getGenericInterfaces() 方法的使用。在此程序中,我们使用了 ArrayList 的类。使用 getGenericInterfaces(),我们检索了所有已实现的接口。
package com.tutorialspoint; import java.lang.reflect.Type; import java.util.ArrayList; public class ClassDemo { public static void main(String []args) { Class c = ArrayList.class; Type[] t = c.getGenericInterfaces(); if(t.length != 0) { for(Type val : t) { System.out.println(val.toString()); } } else { System.out.println("Interfaces are not implemented..."); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
java.util.List<E> interface java.util.RandomAccess interface java.lang.Cloneable interface java.io.Serializable