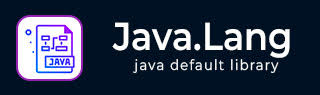
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java 类 getInterfaces() 方法
描述
Java 类 getInterfaces() 方法确定此对象所表示的类或接口实现的接口。
声明
以下是 java.lang.Class.getInterfaces() 方法的声明
public Class<?>[] getInterfaces()
参数
无
返回值
此方法返回一个由该类实现的接口数组。
异常
无
获取实现接口的类的接口示例
以下示例演示了 java.lang.Class.getInterfaces() 方法的用法。在此程序中,我们创建了一个 ClassDemo 的实例,然后使用 getClass() 方法检索实例的类。使用 getInterfaces(),我们检索了所有实现的接口。
package com.tutorialspoint; import java.util.Arrays; interface Print { public void show(); } public class ClassDemo implements Print { public static void main(String[] args) { ClassDemo c = new ClassDemo(); show(c.getClass()); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } public void show(){} }
输出
让我们编译并运行上述程序,这将产生以下结果:
Class = com.tutorialspoint.ClassDemo Classes = [] Interfaces = [interface com.tutorialspoint.Print]
获取 Thread 类接口的示例
以下示例演示了 java.lang.Class.getInterfaces() 方法的用法。在此程序中,我们使用了 Thread 类,然后使用 getInterfaces() 方法检索了所有接口。
package com.tutorialspoint; import java.util.Arrays; public class ClassDemo { public static void main(String[] args) { show(Thread.class); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Class = java.lang.Thread Classes = [interface java.lang.Thread$UncaughtExceptionHandler, class java.lang.Thread$State] Interfaces = [interface java.lang.Runnable]
获取 ArrayList 类接口的示例
以下示例演示了 java.lang.Class.getInterfaces() 方法的用法。在此程序中,我们使用了 Thread 类,然后使用 getInterfaces() 方法检索了所有接口。
package com.tutorialspoint; import java.util.Arrays; public class ClassDemo { public static void main(String[] args) { show(ArrayList.class); } public static void show(Class cls) { System.out.println("Class = " + cls.getName()); Class[] c = cls.getClasses(); System.out.println("Classes = " + Arrays.asList(c)); // returns an array of interfaces Class[] i = cls.getInterfaces(); System.out.println("Interfaces = " + Arrays.asList(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Class = java.util.ArrayList Classes = [] Interfaces = [interface java.util.List, interface java.util.RandomAccess, interface java.lang.Cloneable, interface java.io.Serializable]
java_lang_class.htm
广告