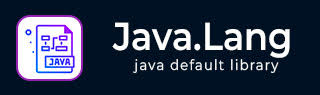
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java ClassLoader getSystemResourceAsStream() 方法
描述
Java ClassLoader getSystemResourceAsStream() 方法用于从用于加载类的搜索路径中打开指定名称的资源以进行读取。
声明
以下是 java.lang.ClassLoader.getSystemResourceAsStream() 方法的声明
public static InputStream getSystemResourceAsStream(String name)
参数
name - 这是资源名称。
返回值
此方法返回用于读取资源的输入流,如果找不到资源则返回 null。
异常
无
使用 ClassLoader 示例获取资源作为流
以下示例演示了 java.lang.ClassLoader.getSystemResourceAsStream() 方法的使用。在此程序中,我们检索了 ClassLoaderDemo 的类。然后使用 getClassLoader() 获取所需的 ClassLoader。现在假设 file.txt 位于 java 程序的类路径中,我们使用 getSystemResourceAsStream() 检索了文件的流内容并相应地打印了内容。
package com.tutorialspoint; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; class ClassLoaderDemo { static String getSystemResource(String rsc) { String val = ""; try { Class cls = Class.forName("com.tutorialspoint.ClassLoaderDemo"); // returns the ClassLoader object associated with this Class ClassLoader cLoader = cls.getClassLoader(); // input stream InputStream inputStream = cLoader.getSystemResourceAsStream(rsc); if(inputStream != null) { BufferedReader buffer = new BufferedReader(new InputStreamReader(inputStream)); // reads each line String l; while((l = buffer.readLine()) != null) { val = val + l; } buffer.close(); inputStream.close(); } } catch(Exception e) { System.out.println(e); } return val; } public static void main(String[] args) { System.out.println("File: " + getSystemResource("file.txt")); } }
输出
假设我们有一个文本文件 file.txt,其内容如下:
This is TutorialsPoint!
让我们编译并运行以上程序,这将产生以下结果:
File: This is TutorialsPoint!
使用 ClassLoader 示例检查资源作为流
以下示例演示了 java.lang.ClassLoader.getSystemResourceAsStream() 方法的使用。在此程序中,我们检索了 ClassLoaderDemo 的类。然后使用 getClassLoader() 获取所需的 ClassLoader。现在假设 test.txt 不在类路径中,我们使用 getSystemResourceAsStream() 获取流为 null 并相应地打印了消息。
package com.tutorialspoint; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; class ClassLoaderDemo { static String getSystemResource(String rsc) { String val = ""; try { Class cls = Class.forName("com.tutorialspoint.ClassLoaderDemo"); // returns the ClassLoader object associated with this Class ClassLoader cLoader = cls.getClassLoader(); // input stream InputStream inputStream = cLoader.getSystemResourceAsStream(rsc); if(inputStream != null) { BufferedReader buffer = new BufferedReader(new InputStreamReader(inputStream)); // reads each line String l; while((l = buffer.readLine()) != null) { val = val + l; } buffer.close(); inputStream.close(); } else{ System.out.println("File not found!"); } } catch(Exception e) { System.out.println(e); } return val; } public static void main(String[] args) { System.out.println("File: " + getSystemResource("test.txt")); } }
输出
假设我们有另一个文本文件 test.txt,但不在类路径中,其内容如下:
This is Java Tutorial
让我们编译并运行以上程序,这将产生以下结果:
File not found! File:
java_lang_classloader.htm
广告