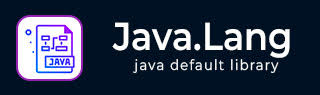
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Long numberOfTrailingZeros() 方法
描述
Java Long numberOfTrailingZeros() 方法返回指定长整型值的二进制补码表示中最低位(“最右边”)1 位之后零位的数量。
如果指定值在其二进制补码表示中没有 1 位,换句话说,如果它等于零,则返回 32。
声明
以下是java.lang.Long.numberOfTrailingZeros() 方法的声明
public static int numberOfTrailingZeros(long i)
参数
i − 这是长整型值。
返回值
此方法返回指定长整型值二进制补码表示中最高位(“最左边”)1 位之前零位的数量,如果该值为零,则返回 32。
异常
无
从正整数中获取尾随零的示例
以下示例演示了如何使用 Long numberOfTrailingZeros() 方法获取最低位 1 位之后零位的数量。我们创建了一个长整型变量并为其分配了一个正长整型值。然后使用 toBinaryString() 方法,我们打印该值的二进制格式。使用 bitCount(),我们打印 1 位的数量。使用 highestOneBit(),我们打印最高位。使用 lowestOneBit(),我们打印最低位,然后使用 numberOfTrailingZeros() 方法打印最高位 1 位之前的零位的值。
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { long i = 170; System.out.println("Number = " + i); /* returns the string representation of the unsigned long value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Long.toBinaryString(i)); // returns the number of one-bits System.out.println("Number of one bits = " + Long.bitCount(i)); /* returns an long value with at most a single one-bit, in the position of the highest-order ("leftmost") one-bit in the specified long value */ System.out.println("Highest one bit = " + Long.highestOneBit(i)); /* returns an long value with at most a single one-bit, in the position of the lowest-order ("rightmost") one-bit in the specified long value.*/ System.out.println("Lowest one bit = " + Long.lowestOneBit(i)); /*returns the number of zero bits following the lowest-order ("rightmost")one-bit */ System.out.print("Number of trailling zeros = "); System.out.println(Long.numberOfTrailingZeros(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = 170 Binary = 10101010 Number of one bits = 4 Highest one bit = 128 Lowest one bit = 2 Number of trailling zeros = 1
从负整数中获取尾随零的示例
以下示例演示了如何使用 Long numberOfTrailingZeros() 方法获取最高位 1 位之前的零位的数量。我们创建了一个长整型变量并为其分配了一个负长整型值。然后使用 toBinaryString() 方法,我们打印该值的二进制格式。使用 bitCount(),我们打印 1 位的数量。使用 highestOneBit(),我们打印最高位。使用 lowestOneBit(),我们打印最低位,然后使用 numberOfTrailingZeros() 方法打印最高位 1 位之前的零位的值。
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { long i = -170; System.out.println("Number = " + i); /* returns the string representation of the unsigned long value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Long.toBinaryString(i)); // returns the number of one-bits System.out.println("Number of one bits = " + Long.bitCount(i)); /* returns an long value with at most a single one-bit, in the position of the highest-order ("leftmost") one-bit in the specified long value */ System.out.println("Highest one bit = " + Long.highestOneBit(i)); /* returns an long value with at most a single one-bit, in the position of the lowest-order ("rightmost") one-bit in the specified long value.*/ System.out.println("Lowest one bit = " + Long.lowestOneBit(i)); /*returns the number of zero bits preceding the highest-order ("leftmost")one-bit */ System.out.print("Number of leading zeros = "); System.out.println(Long.numberOfTrailingZeros(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = -170 Binary = 1111111111111111111111111111111111111111111111111111111101010110 Number of one bits = 60 Highest one bit = -9223372036854775808 Lowest one bit = 2 Number of leading zeros = 1
从正零整数中获取尾随零的示例
以下示例演示了如何使用 Long numberOfTrailingZeros() 方法获取最高位 1 位之前的零位的数量。我们创建了一个长整型变量并为其分配了一个零长整型值。然后使用 toBinaryString() 方法,我们打印该值的二进制格式。使用 bitCount(),我们打印 1 位的数量。使用 highestOneBit(),我们打印最高位。使用 lowestOneBit(),我们打印最低位,然后使用 numberOfTrailingZeros() 方法打印最高位 1 位之前的零位的值。
package com.tutorialspoint; public class LongDemo { public static void main(String[] args) { long i = 0; System.out.println("Number = " + i); /* returns the string representation of the unsigned long value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Long.toBinaryString(i)); // returns the number of one-bits System.out.println("Number of one bits = " + Long.bitCount(i)); /* returns an long value with at most a single one-bit, in the position of the highest-order ("leftmost") one-bit in the specified long value */ System.out.println("Highest one bit = " + Long.highestOneBit(i)); /* returns an long value with at most a single one-bit, in the position of the lowest-order ("rightmost") one-bit in the specified long value.*/ System.out.println("Lowest one bit = " + Long.lowestOneBit(i)); /*returns the number of zero bits preceding the highest-order ("leftmost")one-bit */ System.out.print("Number of leading zeros = "); System.out.println(Long.numberOfTrailingZeros(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = 0 Binary = 0 Number of one bits = 0 Highest one bit = 0 Lowest one bit = 0 Number of trailling zeros = 64