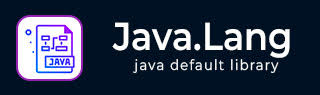
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Integer reverseBytes() 方法
描述
Java Integer reverseBytes() 方法返回通过反转指定 int 值的二进制补码表示中的字节顺序而获得的值。
声明
以下是 java.lang.Integer.reverseBytes() 方法的声明
public static int reverseBytes(int i)
参数
i − 这是 int 值。
返回值
此方法返回通过反转指定 int 值中字节顺序而获得的值。
异常
无
从正整数获取反转位整数示例
以下示例演示了如何使用 Integer reverseBytes() 方法通过反转指定 int 值的二进制补码表示中的字节顺序来获取 int 值。我们创建了一个 int 变量并为其赋值一个正整数。然后使用 toBinaryString() 方法打印值的二进制格式。使用 bitCount() 打印 1 位计数,然后使用 reverseBytes() 方法打印通过反转指定 int 值中字节顺序获得的值。
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { int i = 170; System.out.println("Number = " + i); /* returns the string representation of the unsigned integer value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Integer.toBinaryString(i)); // returns the number of one-bit System.out.println("Number of one bit = " + Integer.bitCount(i)); /* returns the value obtained by reversing order of the bytes in the specified int value */ System.out.println("After reversing = " + Integer.reverseBytes(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = 170 Binary = 10101010 Number of one bit = 4 After reversing = -1442840576
从负整数获取反转位整数示例
以下示例演示了如何使用 Integer reverseBytes() 方法通过反转指定负 int 值的二进制补码表示中的字节顺序来获取 int 值。我们创建了一个 int 变量并为其赋值一个负整数。然后使用 toBinaryString() 方法打印值的二进制格式。使用 bitCount() 打印 1 位计数,然后使用 reverseBytes() 方法打印通过反转指定 int 值中字节顺序获得的值。
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { int i = -170; System.out.println("Number = " + i); /* returns the string representation of the unsigned integer value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Integer.toBinaryString(i)); // returns the number of one-bit System.out.println("Number of one bit = " + Integer.bitCount(i)); /* returns the value obtained by reversing order of the bytes in the specified int value */ System.out.println("After reversing = " + Integer.reverseBytes(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = -170 Binary = 11111111111111111111111101010110 Number of one bit = 28 After reversing = 1459617791
从正零值获取反转位整数示例
以下示例演示了如何使用 Integer reverseBytes() 方法通过反转指定零值的二进制补码表示中的字节顺序来获取 int 值。我们创建了一个 int 变量并为其赋值一个正整数。然后使用 toBinaryString() 方法打印值的二进制格式。使用 bitCount() 打印 1 位计数,然后使用 reverseBytes() 方法打印通过反转指定 int 值中字节顺序获得的值。
package com.tutorialspoint; public class IntegerDemo { public static void main(String[] args) { int i = 0; System.out.println("Number = " + i); /* returns the string representation of the unsigned integer value represented by the argument in binary (base 2) */ System.out.println("Binary = " + Integer.toBinaryString(i)); // returns the number of one-bit System.out.println("Number of one bit = " + Integer.bitCount(i)); /* returns the value obtained by reversing order of the bytes in the specified int value */ System.out.println("After reversing = " + Integer.reverseBytes(i)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Number = 0 Binary = 0 Number of one bit = 0 After reversing = 0