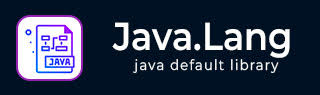
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Number doubleValue() 方法
描述
Java 的 Number doubleValue() 方法用于将任何数字的值转换为其对应的双精度浮点数。
double 是一种基本数据类型,它是 64 位双精度浮点数,根据 IEEE 754 标准,其范围大于 float 数据类型。其取值范围从 1.7976931348623157 x 10308 到 4.9406564584124654 x 10-324(正负)。
double 的默认值和大小分别为 0.0d 和 8 字节。
注意 - 此转换可能涉及给定数字的舍入或截断。
语法
以下是 Java Number doubleValue() 方法的语法
public abstract double doubleValue()
参数
此方法不接受任何参数。
返回值
此方法在转换后返回双精度浮点数。
从整数获取双精度浮点数示例
以下示例演示了 Java Number doubleValue() 方法的使用。在这里,我们正在实例化一个 Integer 对象并对其进行初始化。然后在此对象上调用该方法。获得的返回值将是相应的双精度浮点数。
public class NumberDoubleVal { public static void main(String[] args) { // get a number as integer Integer x = new Integer(123456); // print their value as double System.out.println("x as integer :" + x + ", x as double:" + x.doubleValue()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
x as integer :123456, x as double:123456.0
从整数获取双精度浮点数示例
实现上述示例的另一种方法如下所示。在将整数值赋给 Integer 类引用变量后,创建一个对象。然后在此对象上调用该方法以获取双精度浮点数。
public class NumberDoubleVal { public static void main(String[] args) { // get a number as integer Integer x = 123456; // print their value as double System.out.println("x as integer :" + x + ", x as double:" + x.doubleValue()); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
x as integer :123456, x as double:123456.0
从长整型获取双精度浮点数示例
与前面的示例类似,这里创建了一个 Long 对象,并在其上调用该方法将其转换为双精度浮点数。
public class NumberDoubleVal { public static void main(String[] args) { // get a number as long Long x = (long) 8731029; // print their value as double System.out.println("x as long: " + x + ", x as double: " + x.doubleValue()); } }
输出
上述程序的输出如下所示:
x as long: 8731029, x as double: 8731029.0
示例
以下示例创建一个 Float 对象,并在其上调用该方法。然后,该方法将分配给此对象的值转换为双精度浮点数。
public class NumberDoubleVal { public static void main(String[] args) { // get a number as float Float x = 67929f; // print their value as double System.out.println("x as float: " + x + ", x as double: " + x.doubleValue()); } }
输出
在编译并执行给定程序后,输出将显示如下:
x as float: 67929.0, x as double: 67929.0
从字节获取双精度浮点数示例
在这里,在 Byte 对象上调用该方法,将分配的字节值转换为双精度浮点数。
public class NumberDoubleVal { public static void main(String[] args) { // get a number as byte Byte x = 110; // print their value as double System.out.println("x as byte: " + x + ", x as double: " + x.doubleValue()); } }
输出
编译并运行程序后,输出将打印如下所示:
x as byte: 110, x as double: 110.0
java_lang_number.htm
广告