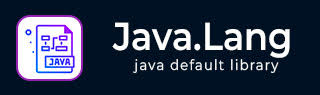
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - Number floatValue() 方法
Java 的 Number floatValue() 方法用于将指定数字的值转换为浮点值。
float 是一种原始数据类型,是单精度 32 位 IEEE 754 浮点值。float 值的范围从 3.40282347 x 1038 到 1.40239846 x 10-45。当需要在存储大量浮点数组时节省内存时,使用此数据类型。
float 的默认值为 0.0f;其默认大小为 4 个字节。
注意 - 此转换可能涉及给定数字的舍入或截断。
语法
以下是 Java Number floatValue() 方法的语法
public abstract float floatValue()
参数
此方法不接受任何参数。
返回值
此方法在转换给定数值后返回浮点值。
从 Integer 获取浮点值示例
以下示例演示了 Java Number floatValue() 方法的用法。整数值被赋给 Integer 类引用变量;并调用在此创建的对象上调用此方法以将整数值转换为浮点值。
package com.tutorialspoint; public class NumberFloatVal { public static void main(String[] args) { // get a number as integer Integer x = 123456; // print their value as float System.out.println("x as integer: " + x + ", x as float: " + x.floatValue()); } }
让我们编译并运行上述程序,这将产生以下结果:
x as integer: 123456, x as float: 123456.0
从 Long 获取浮点值示例
在此示例中,我们首先将长整数值赋给 Long 类引用变量。然后在此创建的 Float 对象上调用此方法,并将长整数值转换为浮点值。
package com.tutorialspoint; public class NumberFloatVal { public static void main(String[] args) { // Get a number as long Long x = (long) 192873; // Print their value as float System.out.println("x as long: " + x + ", x as float: " + x.floatValue()); } }
编译并执行程序后,输出显示为:
x as long: 192873, x as float: 192873.0
从 Double 获取浮点值示例
在这里,与前面的示例类似,Double 类引用变量被赋予一个双精度值。然后在此对象上调用此方法以将其双精度值转换为相应的浮点值。
package com.tutorialspoint; public class NumberFloatVal { public static void main(String[] args) { // get a number as double Double x = 92873d; // print their value as float System.out.println("x as double: " + x + ", x as float: " + x.floatValue()); } }
上述程序的输出如下所示:
x as double: 92873.0, x as float: 92873.0
从 Byte 获取浮点值示例
我们还可以将字节值转换为其对应的双精度值。在创建对象之后,将字节值赋给 Byte 类引用变量。此方法将在此对象上调用,以将字节值转换为双精度值。
package com.tutorialspoint; public class NumberFloatVal { public static void main(String[] args) { // get a number as byte Byte x = -120; // print their value as float System.out.println("x as byte: " + x + ", x as float: " + x.floatValue()); } }
程序编译并运行后,输出显示如下:
x as byte: -120, x as float: -120.0
java_lang_number.htm
广告