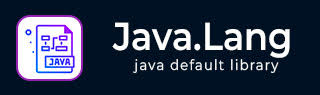
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java 对象 notifyAll() 方法
描述
Java Object notifyAll() 方法唤醒正在等待此对象监视器的所有线程。线程通过调用其中一个 wait 方法来等待对象的监视器。
唤醒的线程在当前线程释放此对象的锁之前将无法继续执行。唤醒的线程将以通常的方式与任何其他可能正在积极竞争同步此对象的线程竞争;例如,唤醒的线程在成为下一个锁定此对象的线程方面没有任何可靠的特权或劣势。
此方法只能由拥有此对象监视器的线程调用。
声明
以下是 java.lang.Object.notifyAll() 方法的声明
public final void notifyAll()
Learn Java in-depth with real-world projects through our Java certification course. Enroll and become a certified expert to boost your career.
参数
无
返回值
此方法不返回值。
异常
IllegalMonitorStateException - 如果当前线程不是此对象监视器的拥有者。
唤醒所有线程的示例
以下示例演示了 java.lang.Object.notifyAll() 方法的用法。在此程序中,我们创建了 ObjectDemo 类,该类具有 addElement 和 removeElement 方法。这些方法本质上是同步的,并且在添加元素时,我们使用 notifyAll() 方法来唤醒所有等待访问对象监视器的线程。在 main 方法中,我们有两个可运行实例,一个用于删除元素,另一个用于添加元素。创建并启动线程后,将调用 addElement() 和 removeElement() 方法。
package com.tutorialspoint; import java.util.Collections; import java.util.LinkedList; import java.util.List; public class ObjectDemo extends Object { private List synchedList; public ObjectDemo() { // create a new synchronized list to be used synchedList = Collections.synchronizedList(new LinkedList()); } // method used to remove an element from the list public String removeElement() throws InterruptedException { synchronized (synchedList) { // while the list is empty, wait while (synchedList.isEmpty()) { System.out.println("List is empty..."); synchedList.wait(); System.out.println("Waiting..."); } String element = (String) synchedList.remove(0); return element; } } // method to add an element in the list public void addElement(String element) { System.out.println("Opening..."); synchronized (synchedList) { // add an element and notify all that an element exists synchedList.add(element); System.out.println("New Element:'" + element + "'"); synchedList.notifyAll(); System.out.println("notifyAll called!"); } System.out.println("Closing..."); } public static void main(String[] args) { final ObjectDemo demo = new ObjectDemo(); Runnable runA = new Runnable() { public void run() { try { String item = demo.removeElement(); System.out.println("" + item); } catch (InterruptedException ix) { System.out.println("Interrupted Exception!"); } catch (Exception x) { System.out.println("Exception thrown."); } } }; Runnable runB = new Runnable() { // run adds an element in the list and starts the loop public void run() { demo.addElement("Hello!"); } }; try { Thread threadA1 = new Thread(runA, "A"); threadA1.start(); Thread.sleep(500); Thread threadA2 = new Thread(runA, "B"); threadA2.start(); Thread.sleep(500); Thread threadB = new Thread(runB, "C"); threadB.start(); Thread.sleep(1000); threadA1.interrupt(); threadA2.interrupt(); } catch (InterruptedException x) { } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
List is empty... List is empty... Opening... New Element:'Hello!' notifyAll called! Closing... Waiting... Waiting... List is empty... Hello! Interrupted Exception!
java_lang_object.htm
广告