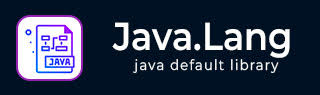
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java 包 getAnnotation() 方法
描述
Java 包 getAnnotation(Class<A> annotationClass) 方法如果存在此元素的指定类型的注释,则返回该元素的注释,否则返回 null。
声明
以下是 java.lang.Package.getAnnotation() 方法的声明
public <A extends Annotation> A getAnnotation(Class<A> annotationClass)
参数
annotationClass − 与注释类型对应的 Class 对象
返回值
如果此元素上存在指定注释类型,则此方法返回此元素的注释,否则返回 null
异常
NullPointerException − 如果给定的注释类为 null
IllegalMonitorStateException − 如果当前线程不是对象监视器的所有者。
获取给定类型的注释示例
以下示例显示了 getAnnotation() 方法的用法。在此程序中,我们已将一个注释 Demo 声明为一个接口,其中包含一个返回字符串的方法和另一个返回整数值的方法。在 PackageDemo 类中,我们在一个名为 example() 的方法上应用了该注释 Demo,并赋予了一些值。在 example() 方法中,我们使用 getClass() 方法检索了 PackageDemo 类的类。
现在使用 getMethod() 示例,我们检索了 example 方法实例,然后使用 getAnnotation() 方法,我们将 Demo 类作为参数传递以获取注释对象。然后使用注释对象获取分配给应用的注释的值。在 main 方法中,调用了此 example() 方法并打印结果。
package com.tutorialspoint; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.reflect.Method; // declare a new annotation @Retention(RetentionPolicy.RUNTIME) @interface Demo { String str(); int val(); } public class PackageDemo { // set values for the annotation @Demo(str = "Demo Annotation", val = 100) // a method to call in the main public static void example() { PackageDemo ob = new PackageDemo(); try { Class c = ob.getClass(); // get the method example Method m = c.getMethod("example"); // get the annotation for class Demo Demo annotation = m.getAnnotation(Demo.class); // print the annotation System.out.println(annotation.str() + " " + annotation.val()); } catch (NoSuchMethodException exc) { exc.printStackTrace(); } } public static void main(String args[]) { example(); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Demo Annotation 100
获取给定类型的注释时遇到异常的示例
以下示例显示了 getAnnotation() 方法的用法。在此程序中,我们已将一个注释 Demo 声明为一个接口,其中包含一个返回字符串的方法和另一个返回整数值的方法。在 PackageDemo 类中,我们在一个名为 example() 的方法上应用了该注释 Demo,并赋予了一些值。在 example() 方法中,我们使用 getClass() 方法检索了 PackageDemo 类的类。
现在使用 getMethod() 示例,我们检索了 example 方法实例,然后使用 getAnnotation() 方法,我们将 null 作为参数传递以获取注释对象。由于这会导致 NullPointerException,因此当程序执行时,异常处理程序块会打印错误消息。
package com.tutorialspoint; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.reflect.Method; // declare a new annotation @Retention(RetentionPolicy.RUNTIME) @interface Demo { String str(); int val(); } public class PackageDemo { // set values for the annotation @Demo(str = "Demo Annotation", val = 100) // a method to call in the main public static void example() { PackageDemo ob = new PackageDemo(); try { Class c = ob.getClass(); // get the method example Method m = c.getMethod("example"); // get the annotation Demo annotation = m.getAnnotation(null); if(annotation != null) { // print the annotation System.out.println(annotation.str() + " " + annotation.val()); } } catch (Exception e) { e.printStackTrace(); } } public static void main(String args[]) { example(); } }
输出
让我们编译并运行以上程序,这将产生以下结果:
java.lang.NullPointerException at java.base/java.util.Objects.requireNonNull(Objects.java:233) at java.base/java.lang.reflect.Executable.getAnnotation(Executable.java:599) at java.base/java.lang.reflect.Method.getAnnotation(Method.java:793) at com.tutorialspoint.PackageDemo.example(PackageDemo.java:29) at com.tutorialspoint.PackageDemo.main(PackageDemo.java:40)