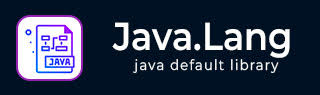
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java 包 getAnnotations() 方法
描述
Java 包 getAnnotations() 方法返回此元素上存在的所有注释。(如果此元素没有注释,则返回长度为零的数组。)此方法的调用者可以自由修改返回的数组;这不会影响返回给其他调用者的数组。
声明
以下是java.lang.Package.getAnnotations() 方法的声明
public Annotation[] getAnnotations()
参数
无
返回值
此方法返回此元素上存在的所有注释
异常
无
获取所有注释示例
以下示例演示了 getAnnotations() 方法的用法。在这个程序中,我们声明了一个注释 Demo 作为接口,它包含一个返回字符串的方法和另一个返回整数值的方法。在 PackageDemo 类中,我们在一个名为 example() 的方法上应用了该 Demo 注释,并赋予了一些值。在 example() 方法中,我们使用 getClass() 方法检索了 PackageDemo 类的类。
现在使用 getMethod() 示例,我们检索 example 方法实例,然后使用 getAnnotations() 方法检索注释数组并打印它们。在主方法中,调用此 example() 方法并打印结果。
package com.tutorialspoint; import java.lang.annotation.Annotation; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.reflect.Method; // declare a new annotation @Retention(RetentionPolicy.RUNTIME) @interface Demo { String str(); int val(); } public class PackageDemo { // set values for the annotation @Demo(str = "Demo Annotation", val = 100) // a method to call in the main public static void example() { PackageDemo ob = new PackageDemo(); try { Class c = ob.getClass(); // get the method example Method m = c.getMethod("example"); // get the annotations Annotation[] annotation = m.getAnnotations(); // print the annotation for (int i = 0; i < annotation.length; i++) { System.out.println(annotation[i]); } } catch (NoSuchMethodException exc) { exc.printStackTrace(); } } public static void main(String args[]) { example(); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
@com.tutorialspoint.Demo(str=Demo Annotation, val=100)
获取空注释数组示例
以下示例演示了 getAnnotations() 方法的用法。在这个程序中,我们声明了一个注释 Demo 作为接口,它包含一个返回字符串的方法和另一个返回整数值的方法。在 PackageDemo 类中,我们在一个名为 example() 的方法上应用了该 Demo 注释,并赋予了一些值。在 example() 方法中,我们使用 getClass() 方法检索了 PackageDemo 类的类。我们创建了另一个名为 example1() 的方法,该方法没有应用任何注释。
现在使用 getMethod() 示例,我们检索 example1 方法实例,然后使用 getAnnotations() 方法检索注释数组并打印它们。由于没有应用任何注释,因此数组将为空。在主方法中,调用此 example() 方法并打印结果。
package com.tutorialspoint; import java.lang.annotation.Annotation; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.reflect.Method; // declare a new annotation @Retention(RetentionPolicy.RUNTIME) @interface Demo { String str(); int val(); } public class PackageDemo { // set values for the annotation @Demo(str = "Demo Annotation", val = 100) // a method to call in the main public static void example() { PackageDemo ob = new PackageDemo(); try { Class c = ob.getClass(); // get the method example Method m = c.getMethod("example1"); // get the annotations Annotation[] annotation = m.getAnnotations(); if(annotation.length != 0) { // print the annotation for (int i = 0; i < annotation.length; i++) { System.out.println(annotation[i]); } }else { System.out.println("No annotations present."); } } catch (NoSuchMethodException exc) { exc.printStackTrace(); } } public static void main(String args[]) { example(); } public static void example1() { // method with no annotations } }
输出
让我们编译并运行上述程序,这将产生以下结果:
No annotations present.