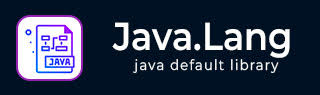
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java Runtime exec() 方法
描述
Java Runtime exec(String[] cmdarray, String[] envp) 方法在单独的进程中使用指定的环境执行指定的命令和参数。这是一种方便的方法。形式为 exec(cmdarray, envp) 的调用行为与调用 exec(cmdarray, envp, null) 完全相同。
声明
以下是java.lang.Runtime.exec()方法的声明
public Process exec(String[] cmdarray, String[] envp)
参数
cmdarray - 包含要调用的命令及其参数的数组。
envp - 字符串数组,每个元素都以 name=value 的格式设置环境变量,或者如果子进程应该继承当前进程的环境则为 null。
返回值
此方法返回一个新的 Process 对象,用于管理子进程
异常
SecurityException - 如果存在安全管理器并且其 checkExec 方法不允许创建子进程
IOException - 如果发生 I/O 错误
NullPointerException - 如果 command 为 null
IndexOutOfBoundsException - 如果 cmdarray 是一个空数组(长度为 0)
示例
此示例需要一个名为example.txt的文件,该文件位于我们的 CLASSPATH 中,内容如下:
Hello World!
示例:使用给定文本文件打开记事本应用程序
以下示例显示了 Java Runtime exec() 方法的用法。在此程序中,我们有一个字符串数组。添加了 Notepad.exe 和 test.txt 条目。我们使用 exec() 方法为记事本可执行文件创建了一个 Process 对象。这将调用记事本应用程序,并将打开 test.txt。如果发生某些异常,则会打印相应的堆栈跟踪以及错误消息。如果 test.txt 不存在于当前类路径中,则记事本将报告不存在文件的错误。
package com.tutorialspoint; public class RuntimeDemo { public static void main(String[] args) { try { // create a new array of 2 strings String[] cmdArray = new String[2]; // first argument is the program we want to open cmdArray[0] = "notepad.exe"; // second argument is a txt file we want to open with notepad cmdArray[1] = "example.txt"; // print a message System.out.println("Executing notepad.exe and opening example.txt"); // create a process and execute cmdArray and currect environment Process process = Runtime.getRuntime().exec(cmdArray,null); // print another message System.out.println("example.txt should now open."); } catch (Exception ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Executing notepad.exe and opening example.txt example.txt should now open.
示例:打开计算器应用程序
以下示例显示了 Java Runtime exec() 方法的用法。在此程序中,我们有一个字符串数组。添加了 calc.exe 条目。我们使用 exec() 方法为计算器可执行文件创建了一个 Process 对象。这将调用计算器应用程序。如果发生某些异常,则会打印相应的堆栈跟踪以及错误消息。
package com.tutorialspoint; public class RuntimeDemo { public static void main(String[] args) { try { // create a new array of 1 string String[] cmdArray = new String[1]; // first argument is the program we want to open cmdArray[0] = "calc.exe"; // print a message System.out.println("Executing calc.exe"); // create a process and execute cmdArray and currect environment Process process = Runtime.getRuntime().exec(cmdArray,null); } catch (Exception ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Executing calc.exe
示例:打开 Windows 资源管理器应用程序
以下示例显示了 Java Runtime exec() 方法的用法。在此程序中,我们有一个字符串数组。添加了 explorer.exe 条目。我们使用 exec() 方法为计算器可执行文件创建了一个 Process 对象。这将调用 Windows 资源管理器应用程序。如果发生某些异常,则会打印相应的堆栈跟踪以及错误消息。
package com.tutorialspoint; public class RuntimeDemo { public static void main(String[] args) { try { // create a new array of 1 string String[] cmdArray = new String[1]; // first argument is the program we want to open cmdArray[0] = "explorer.exe"; // print a message System.out.println("Executing explorer.exe"); // create a process and execute cmdArray and currect environment Process process = Runtime.getRuntime().exec(cmdArray,null); } catch (Exception ex) { ex.printStackTrace(); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
Executing explorer.exe