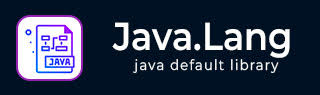
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Short compareTo() 方法
Java Short compareTo() 方法用于按数值比较两个 Short 对象。
当我们需要比较两个给定的 Short 对象的值以检查这两个给定的值是否相等、小于或大于时,可以使用此方法。
在对象中,Short 类封装了 short 类型。short 原生数据类型是一个 16 位带符号的二进制补码整数,其最大值为 32,767,最小值为 -32,768(包含)。
语法
以下是Java Short compareTo()方法的语法:
public int compareTo(Short anotherShort)
参数
anotherShort: 要比较的 short 值。
返回值
此方法返回以下值:
0 如果 anotherShort 在数值上等于此 short。
小于 0 的值,如果此 short 在数值上小于 anotherShort。
大于 0 的值,如果此 short 在数值上大于 anotherShort。
比较包含正 Short 值的两个 Short 包装对象示例
当我们将两个 short 值传递给此方法时,如果第一个值小于第二个值,则返回小于 0 的值。
以下示例显示了 Java Short compareTo() 方法的使用。在这里,创建了两个 Short 对象“obj1”和“obj2”。将值分配给这两个给定的对象,使得第一个值小于第二个值。然后在比较这两个值后检索结果:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // compares two Short objects numerically Short obj1 = new Short("987"); Short obj2 = new Short("7676"); int retval = obj1.compareTo(obj2); if (retval > 0) { System.out.println("obj1 is greater than obj2"); } else if (retval < 0) { System.out.println("obj1 is less than obj2"); } else { System.out.println("obj1 is equal to obj2"); } } }
输出
让我们编译并运行以上程序,这将产生以下结果:
obj1 is less than obj2
比较包含正 Short 值的两个 Short 包装对象示例
当我们将多个整数作为参数传递给此方法时,如果第一个值小于第二个值,则返回小于 0 的值;如果第一个值大于第二个值,则返回大于 0 的值;如果两个值相等,则返回 0。
在以下示例中,创建了三个名为 Shortval1、Shortval2 和 Shortval3 的 Short 对象。然后将这些对象分别赋值为 '50'、'200'、'50' short 变量。然后在对象上调用 compareTo() 方法以在不同的给定情况下比较所有对象:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // create short object and assign value to it short val1 = 50, val2 = 200, val3 = 50; Short Shortval1 = new Short(val1); Short Shortval2 = new Short(val2); Short Shortval3 = new Short(val3); // returns less than 0 if this Short is less than the argument Short int cmp = Shortval1.compareTo(Shortval2); System.out.println("" + Shortval1 + " is less than " + Shortval2 + ",difference = " + cmp); // returns 0 if this Short is equal to the argument Short cmp = Shortval1.compareTo(Shortval3); System.out.println("" + Shortval1 + " is equal to " + Shortval3 + ",difference = " + cmp); // returns greater than if this Short is greater than the argument Short cmp = Shortval2.compareTo(Shortval1); System.out.println("" + Shortval2 + " is more than " + Shortval1 + ",difference = " + cmp); } }
输出
以下是上述代码的输出:
50 is less than 200, difference = -150 50 is equal to 50, difference = 0 200 is more than 50, difference = 150
比较包含负 Short 值的两个 Short 包装对象示例
当我们使用此方法创建一个 Short 对象数组时,分别与数组中的第一个元素进行比较,将返回大于 0、小于 0 和等于 0 的值。
在此示例中,创建了一个 short 类型的数组,并且通过分配一个 for 循环,数组中的每个对象都与数组的第一个对象进行比较:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short[] object = { -854, -546, -854, -917 }; for (int i = 0; i < 4; i++) { // comparing every object in the array with the first object System.out.println(object[0].compareTo(object[i])); } } }
输出
在执行上述程序后,获得的输出如下:
0 -308 0 63