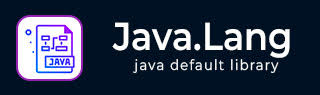
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Short equals() 方法
Java Short equals() 方法用于比较两个给定值的相等性。此方法返回一个布尔值。
在数学中,等式是一种关系,它声明两个量具有相同的值,或者两个数学表达式反映相同的数学对象。x 和 y 之间的等式表示为 x=y。
语法
以下是Java Short equals() 方法的语法:
public boolean equals(Object obj)
参数
obj − 这是要比较的对象。
Learn Java in-depth with real-world projects through our Java certification course. Enroll and become a certified expert to boost your career.
返回值
如果对象相同,则此方法返回 true,否则返回 false。
比较具有相同 short 值的 Short 对象的相等性示例
如果我们通过此方法将两个彼此相似的对象作为参数传递,则它将返回 true。
以下示例演示了使用 Java Short equals() 方法将此对象与指定对象进行比较。创建了两个 Short 对象“obj1”和“obj2”。然后将值分配给这些对象,这些值彼此相等。此后,使用 equals() 方法检查值的相等性:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // compares this object against the specified object Short obj1 = new Short("2"); Short obj2 = new Short("02"); System.out.print(obj1 + " = " + obj2); System.out.println(" ? " + obj1.equals(obj2)); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
2 = 2 ? true
比较具有不同 short 值的 Short 对象的相等性示例
当我们通过此方法将两个具有不同值的作为参数传递时,将返回 false。
在下面的示例中,创建了两个 Short 对象“obj1”和“obj2”。然后将两个不同的值分配给这些对象以检查它们的相等性:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // compares this object against the specified object Short obj1 = new Short("87"); Short obj2 = new Short("45"); System.out.print(obj1 + " = " + obj2); System.out.println(" ? " + obj1.equals(obj2)); } }
输出
以下是上述代码的输出:
87 = 45 ? false
比较具有 short 值的 Short 对象的相等性示例
以下是一个示例,其中创建了两个 Short 对象,并将值分配给这些对象。然后使用 if-else 语句比较这些值。然后返回相应的结果:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // same objects Short value1 = new Short("2345"); Short value2 = new Short("2345"); System.out.print("obj1 & obj2: "); if (value1.equals(value2)) System.out.println("The given two objects are same"); else System.out.println("The given two objects are not same"); // different objects value1 = new Short("4567"); value2 = new Short("789"); System.out.print("obj1 & obj2: "); if (value1.equals(value2)) System.out.println("The given two objects are same"); else System.out.println("The given two objects are not same"); } }
输出
执行上述程序后,获得的输出如下:
obj1 & obj2: The given two objects are same obj1 & obj2: The given two objects are not same
比较 Short 对象的相等性示例
以下示例演示了使用 Java Short equals() 方法将此对象与指定对象进行比较。
package com.tutorialspoint; import java.util.Scanner; public class ShortDemo { public static void main(String[] args) { Short obj = new Short("3"); System.err.println( "Question: What is the full form of SIM ?\n 1.Subscriber's Identity Machine \b 2.Self Identity Machine \b 3.Subscriber's Identity Module"); Scanner scanner = new Scanner(System.in); Short b = 3; if (b.equals(obj)) { System.out.println("Hurray! You got the correct answer!"); } else { System.out.println("opps! Your answer is incorrect!"); } b = 2; if (b.equals(obj)) { System.out.println("Hurray! You got the correct answer!"); } else { System.out.println("opps! Your answer is incorrect!"); } } }
输出
执行上述代码时,我们将获得以下输出:
Question: What is the full form of SIM ? 1.Subscriber's Identity Machine 2.Self Identity Machine 3.Subscriber's Identity Module Hurray! You got the correct answer! opps! Your answer is incorrect!