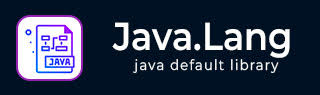
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Short floatValue() 方法
**Java Short floatValue() 方法** 在进行扩展原始类型转换(将较小的数据类型转换为较大的数据类型)后,检索给定 short 值的等效 float 值。float 数据类型可以存储范围在 3.4e-038 到 3.4e+038 之间的分数。
这可能涉及舍入或截断。在 Java 编程中,截断是指从字符串右侧删除某些 float 或 double 类型数字。舍入是指将给定值四舍五入到其最接近的 float 值。
语法
以下是 **Java Short floatValue()** 方法的语法:
public float floatValue()
参数
此方法不接受任何参数。
返回值
此方法在转换为 float 类型后,返回此对象表示的数值。
从具有正值的 Short 获取 float 值示例
以下示例演示了通过创建 Short 对象 'obj' 并为其赋值来使用 Java Short floatValue() 方法。此后,通过在 Short 对象上调用该方法来返回 short 的等效 float 值:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // returns the float value of this Short object Short obj = new Short("5"); float f = obj.floatValue(); System.out.println("Value = " + f); obj = new Short("32"); f = obj.floatValue(); System.out.println("Value = " + f); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Value = 5.0 Value = 32.0
从具有负值的 Short 获取 float 值示例
以下是 Java Short floatValue() 方法的一个示例。在这里,我们将负 short 值分配给创建的 Short 对象“obj”,并打印其等效的 float 值:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short obj = new Short("-65"); float f = obj.floatValue(); System.out.println("Value = " + f); } }
输出
以下是上述代码的输出:
Value = -65.0
从具有正值的 Short 获取 float 值示例
在以下示例中,创建了一个值为“8986”的 Short 对象,并使用 Java Short floatValue() 方法将其转换为 float:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { short obj = 8986; Short s = new Short(obj); System.out.println("The Short value is = " + s); // Short into float conversion float f = s.floatValue(); System.out.println("The converted Float value is = " + f); } }
输出
在执行上述程序时,将获得如下输出:
The Short value is = 8986 The converted Float value is = 8986.0
从具有正值的 Short 获取 float 值示例
以下是 floatValue() 方法的另一个示例:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // initializing the variables short value1 = 76; short value2 = 87; // Returning the short value represented by this Short object 'res1' // and converting it to a float by calling res1.floatValue() Short res1 = new Short(value1); // Printing the res1 result System.out.println("The float value of res1 is: " + res1.floatValue()); // Returning the short value represented by this Short object 'res1' // and converting it to a float by calling res2.floatValue() Short res2 = new Short(value2); // Printing the res2 result System.out.println("The float value of res2 is: " + res2.floatValue()); } }
输出
在执行上述代码时,我们得到以下输出:
The float value of res1 is: 76.0 The float value of res2 is: 87.0
java_lang_short.htm
广告