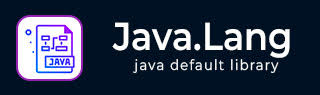
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Short intValue() 方法
Java Short intValue() 方法在进行扩展基本类型转换(将较小的数据类型转换为较大的数据类型)后,检索给定 short 值的等效整数值。它是 Number 类的继承成员。int 数据类型可以在 -2,147,483,648 到 2,147,483,647 的范围内存储整数。
简而言之,使用此方法,Short 对象值将转换为基本整数值。
例如,假设我们有一个 short 值“87”。给定 short 值的 int 值为“87”。
Short d1 = 87 Int value = 87
语法
以下是Java Short intValue()方法的语法:
public int intValue()
参数
此方法不接受任何参数。
返回值
此方法返回此对象表示的 short 值转换为 int 类型。
从正值 Short 获取 int 值示例
以下是一个示例,演示 Java Short intValue() 方法的使用。在这里,我们使用正值创建一个 Short 对象。然后,打印它的整数值:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { /* * returns the short value represented by this object * converted to type int */ Short obj = new Short("32"); int i = obj.intValue(); System.out.println("Value = " + i); obj = new Short("30"); i = obj.intValue(); System.out.println("Value = " + i); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Value = 32 Value = 30
从 Short 对象获取 int 值示例
以下是此函数的另一个示例:
package com.tutorialspoint; import java.util.Scanner; public class ShortDemo { public static void main(String[] args) { Short value1 = -35; Short value2 = -84; Short value3 = 987; System.out.println("Product of negative numbers = " + value1 * value2); System.out.println("Product of negative numbers = " + value1 * value3); int s = value1.intValue() * value2.intValue(); System.out.println("Product of integer values = " + s); } }
输出
以下是上述代码的输出:
Product of negative numbers = 2940 Product of negative numbers = -34545 Product of integer values = 2940
从具有最大值的 Short 对象获取 int 值示例
在下面的示例中,创建了 short 变量“value”。然后将 MAX_VALUE 分配给此变量。然后,我们检索其对应的整数值:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short value = Short.MAX_VALUE; System.out.println("The short MAX_VALUE is: " + value); // it returns int type value int result = value.intValue(); System.out.println("The int value of short is: " + result); } }
输出
在执行上述程序后,输出如下:
The short MAX_VALUE is: 32767 The int value of short is: 32767
从具有最小值的 Short 对象获取 int 值示例
在下面的示例中,创建了 short 变量“value”。然后将 MIN_VALUE 分配给此变量。然后,我们检索其对应的整数值:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short value = Short.MIN_VALUE; System.out.println("The short MIN_VALUE is: " + value); // it returns int type value int result = value.intValue(); System.out.println("The int value of short is: " + result); } }
输出
在执行上述代码时,我们得到以下输出:
The short MIN_VALUE is: -32768 The int value of short is: -32768
java_lang_short.htm
广告