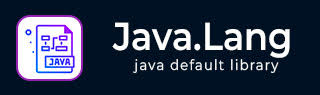
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - Short parseShort() 方法
Java Short parseShort() 方法用于将给定的字符串值解析(转换)为 Short 对象。此方法将给定的字符串解析为带符号的十进制短整型。
提供的字符串中的字符必须全部为十进制数字,除了第一个字符,它可以是 ASCII 减号 ('-') 或 ASCII 加号 ('+') ('u002B'),分别表示负值或正值。
语法
以下是 Java Short parseShort() 方法的语法:-
public static short parseShort(String s) throws NumberFormatException or, public static short parseShort(String s, int radix) throwsNumberFormatException
参数
s - 这是一个包含要解析的短整型表示形式的字符串。
radix - 这是解析 s 时使用的基数。
Learn Java in-depth with real-world projects through our Java certification course. Enroll and become a certified expert to boost your career.
返回值
此方法返回由字符串参数在指定基数中表示的短整型值。
从包含正值的字符串获取短整型值示例
我们可以使用 parseShort() 方法传递正值。结果将是给定字符串值的等效短整型值。
以下示例显示了 Java Short parseShort() 方法的使用。这里我们通过创建一个 Short 对象 's' 并返回字符串变量 'str' 表示的短整型值。
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short s = new Short("6"); // returns the short value represented by the string argument String str = "50"; short retval = s.parseShort(str); System.out.println("Value = " + retval); } }
输出
让我们编译并运行上述程序,这将产生以下结果:-
Value = 50
从包含正值的字符串获取短整型值示例
在下面给出的示例中,声明了三个字符串变量,即 's1'、's2'、's3',并为其分配了值。通过使用 parseShort() 方法,传递了与字符串相对应的短整型值以及一些操作,如连接、加法、乘法和除法。然后返回其对应的短整型值:-
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { String s1 = "8"; String s2 = "13"; String s3 = "4"; // concatenating two strings instead of adding it Short d1 = Short.parseShort(s1 + s2 + s3); System.out.println("The sum is =" + d1); System.out.println("The sum is =" + (Short.parseShort(s1) + Short.parseShort(s2) + Short.parseShort(s3))); System.out.println("The Product is =" + (Short.parseShort(s1) * Short.parseShort(s2) * Short.parseShort(s3))); System.out.println("The division is =" + (Short.parseShort(s2) / Short.parseShort(s3))); System.out.println("The division =" + (Short.parseShort(s1) / Short.parseShort(s2))); } }
输出
以下是上述代码的输出:-
The sum is =8134 The sum is =25 The Product is =416 The division is =3 The division =0
从包含无效值的字符串获取短整型值时遇到异常示例
当将字母数字值作为参数传递给此方法时,它会返回 NumberFormat Exception。
在下面的代码中,传递的字符串值不包含可解析的值,因此它会抛出异常错误:-
package com.tutorialspoint; import java.util.Scanner; public class ShortDemo { public static void main(String[] args) { String s = "Deepak@21"; Short d = Short.parseShort(s); System.out.println("The equivalent short value is = " + d); } }
NumberFormatException
上述代码抛出异常错误,如下面的输出所示:-
Exception in thread "main" java.lang.NumberFormatException: For input string: "Deepak@21" at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67) at java.base/java.lang.Integer.parseInt(Integer.java:668) at java.base/java.lang.Short.parseShort(Short.java:137) at java.base/java.lang.Short.parseShort(Short.java:163) at com.tutorialspoint.ShortDemo.main(ShortDemo.java:6)
从具有给定基数的字符串获取短整型值示例
以下是一个示例,其中检索了指定字符串(带基数)的带符号十进制短整型值:-
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { String str = "1000"; // returns signed decimal short value of string short shortValue = Short.parseShort(str); // prints signed decimalshort value System.out.println("Signed decimal short value for given String is = " + shortValue); // returns the string argument as a signed short in the radix shortValue = Short.parseShort(str,2); System.out.println("Signed decimal short value for specified String with radix 2 is = " + shortValue); // returns the string argument as a signed short in the radix shortValue = Short.parseShort("11",8); System.out.println("Signed decimal short value for specified String with radix 8 is = " + shortValue); } }
输出
执行上述代码时,我们将获得以下输出:-
Signed decimal short value for given String is = 1000 Signed decimal short value for specified String with radix 2 is = 8 Signed decimal short value for specified String with radix 8 is = 9