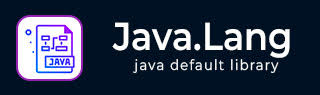
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - 简短的 toString() 方法
Java Short toString() 方法检索给定短整数值的字符串表示形式。该值被转换为带符号十进制数,并作为字符串返回。
Java 中的 Short 对象是“Short”包装类的实例,它存储短整型原始数据类型。但是,在某些情况下,我们需要将 short 转换为 String,因为这允许我们存储 short 或其他存储数字的数据类型(例如整数或浮点数)无法存储的更大数字。
此方法有两个多态变体;无参数和带 short 参数(在下面的语法中讨论)。
语法
以下是Java Short toString() 方法的语法:
public String toString() // without argument or, public static String toString(short s) // with a short argument
参数
s − 这是要转换的 short 值。// 第二种语法
返回值
此方法返回此对象或参数的以 10 为基数的字符串表示形式。(如果传递)。
获取带正值的 Short 的字符串表示形式示例
下面给出的代码演示了 Java Short toString() 方法的使用方法,方法是创建一个 Short 对象。然后返回表示此 short 值的字符串对象,无需传递任何参数:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { Short s = new Short("53"); // returns a string representation String retval = s.toString(); System.out.println("Value = " + retval); } }
输出
让我们编译并运行上述程序,这将产生以下结果:
Value = 53
获取带正值的 Short 的字符串表示形式示例
以下示例使用 try-catch 语句将 short 值赋值给字符串对象 'str',从而将其转换为等效的字符串值:
package com.tutorialspoint; import java.util.Scanner; public class ShortDemo { public static void main(String[] args) { try { Short value1 = 98; // conversion to string String str = value1.toString(); System.out.println("The equivalent string value is = " + str); } catch (Exception e) { System.out.println("Error: Invalid input!"); } try { Short value2 = 0 * 64576; // conversion to string String str2 = value2.toString(); System.out.println("The equivalent string value is = " + str2); } catch (Exception e) { System.out.println("Error: Invalid input!"); } } }
输出
以下是上述代码的输出:
The equivalent string value is = 98 The equivalent string value is = 0
获取带正值的 Short 的字符串表示形式示例
以下是此方法的另一个示例:
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // create short object and assign value to it short sval = 50; Short shortValue = new Short(sval); // returns a String object representing this Short value. System.out.println("Value is = " + shortValue.toString()); // returns a String object representing the specified short System.out.println("Value of specified short is = " + Short.toString((short)sval)); } }
输出
上述代码的输出如下:
Value is = 50 Value of specified short is = 50
获取带负值的 Short 的字符串表示形式示例
在下面的示例中,我们也可以在一个具有负值的 Short 对象上调用 toString() 方法。
package com.tutorialspoint; public class ShortDemo { public static void main(String[] args) { // create short object and assign value to it short sval = -50; Short shortValue = new Short(sval); // returns a String object representing this Short value. System.out.println("Value is = " + shortValue.toString()); } }
输出
执行上述代码后,我们将得到以下输出:
Value is = -50
java_lang_short.htm
广告