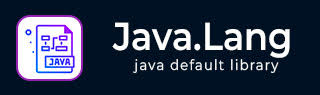
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java StackTraceElement toString() 方法
描述
Java StackTraceElement toString() 方法返回此堆栈跟踪元素的字符串表示形式。
声明
以下是 java.lang.StackTraceElement.toString() 方法的声明
public String toString()
参数
无
返回值
此方法返回对象的字符串表示形式。
异常
无
示例:使用当前线程获取 StackTraceElement 字符串表示形式
以下示例演示了 Java StackTraceElement toString() 方法的使用。在这个程序中,我们定义了一个名为 function2() 的函数,它获取当前线程 StackTraceElement 的字符串表示形式。另一个名为 function1() 的函数用于实例化 StackTraceElementDemo 类并调用 function2() 方法。在 main 方法中,我们调用了 function1() 方法,并打印结果。
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { function1(); } public static void function1() { new StackTraceElementDemo().function2(); } public void function2() { int i; // print stack trace for(i = 1 ; i < 3 ; i++ ) { System.out.println(Thread.currentThread().getStackTrace()[i]. toString()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
com.tutorialspoint.StackTraceElementDemo.function2(StackTraceElementDemo.java:20) com.tutorialspoint.StackTraceElementDemo.function1(StackTraceElementDemo.java:12)
示例:获取 ArithmeticException 的 StackTraceElement 的字符串表示形式
以下示例演示了 Java StackTraceElement toString() 方法的使用。在 main 方法中,我们创建了三个 int 变量,其中一个变量的值为零。在 try-catch 块中,我们创建了一个除以零的情况以引发 ArithmeticException。在 catch 块中,我们处理了 ArithmeticException,使用 getStackTrace() 方法从中检索 StackTraceElement 数组,然后对数组的第一个元素使用 toString() 方法,并打印字符串表示形式。
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(ArithmeticException e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the string representation System.out.println(stackTraceElements[0].toString()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
com.tutorialspoint.StackTraceElementDemo.main(StackTraceElementDemo.java:12)
示例:获取 Exception 的 StackTraceElement 的字符串表示形式
以下示例演示了 Java StackTraceElement toString() 方法的使用。在 main 方法中,我们创建了三个 int 变量,其中一个变量的值为零。在 try-catch 块中,我们创建了一个除以零的情况以引发 ArithmeticException。在 catch 块中,我们处理了 Exception,使用 getStackTrace() 方法从中检索 StackTraceElement 数组,然后对数组的第一个元素使用 toString() 方法,并打印字符串表示形式。
package com.tutorialspoint; public class StackTraceElementDemo { public static void main(String[] args) { // variables to store int values int a = 0, b = 1, c; try { // being divide by zero, this line will throw an ArithmeticException c = b / a; }catch(Exception e) { // get the array of StackTraceElement StackTraceElement[] stackTraceElements = e.getStackTrace(); // print the string representation System.out.println(stackTraceElements[0].toString()); } } }
输出
让我们编译并运行上述程序,这将产生以下结果:
com.tutorialspoint.StackTraceElementDemo.main(StackTraceElementDemo.java:12)