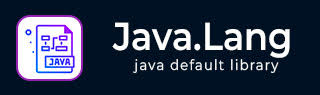
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - String charAt() 方法
描述
Java String charAt() 方法用于检索指定索引处的字符。索引指的是字符在序列中的位置。第一个字符值表示第 0 个索引,下一个字符值表示第 1 个索引,以此类推。索引从 0 开始,到 length()-1 结束。
字符串是一个保存字符序列的对象。java.lang.string 类表示 Java 中的字符串。Java 中的字符串是常量,创建后其值无法更改。
charAt() 方法接受一个整数参数,该参数保存索引的值。如果索引值为负数或大于序列长度,则抛出异常。
语法
以下是Java String charAt() 方法的语法:
public char charAt(int index)
参数
- index - 这是字符值索引。
返回值
此方法返回此字符串中指定索引处的字符值。第一个字符值位于索引 0 处。
从字符串中获取给定索引处的字符示例
如果索引值非负且小于字符串长度,则charAt() 方法返回指定索引处的字符值。
在以下程序中,我们创建一个值为“This is tutorialspoint”的字符串字面量。然后使用charAt() 方法,我们正在检索指定索引0、4 和 17处的字符。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { //create an string literal String str = "This is tutorialspoint"; System.out.print("The string is: " + str); //initialize the index values int index1 = 0; int index2 = 4; int index3 = 17; System.out.println("The index values are: " + index1 + ", " + index2 + " and " + index3); //using the charAt() method System.out.println("The character value at the " + index1 + " index is: " + str.charAt(index1)); System.out.println("The character value at the " + index2 + " index is: " + str.charAt(index2)); System.out.println("The character value at the " + index3 + " index is: " + str.charAt(index3)); } }
输出
执行上述程序后,将产生以下结果:
The string is: This is tutorialspoint The index values are: 0, 4 and 17 The character value at the 0 index is: T The character value at the 4 index is: The character value at the 17 index is: p
从字符串中获取负数索引处的字符时出现异常示例
如果索引值为负数,则此方法会抛出IndexOutOfBoundsException异常。
在此程序中,我们正在创建一个值为“Tutorials Point”的字符串字面量。然后,使用charAt() 方法,我们尝试检索指定索引 -1处的字符值。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { try { // create a string literal String str = "Tutorials Point"; System.out.println("The string value is: " + str); //initialize the index value int index = -1; System.out.println("The index value is: " + index); // use charAt() method System.out.println("The character value at the specifed index " + index + " is: " + str.charAt(index)); } catch(Exception e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The string value is: Tutorials Point The index value is: -1 java.lang.StringIndexOutOfBoundsException: String index out of range: -1 at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48) at java.base/java.lang.String.charAt(String.java:1515) at com.tutorialspoint.Demo.main(Demo.java:13) Exception: java.lang.StringIndexOutOfBoundsException: String index out of range: -1
从字符串中获取无效索引处的字符示例
如果索引值大于字符串长度,则CharAt() 方法会抛出IndexOutOfBoundsException异常。
在以下示例中,我们使用值为"Welcome to Tutorials Point"创建字符串类的一个对象。然后,使用charAt() 方法,我们尝试检索指定索引处的字符,其值大于字符串长度。
package com.tutorialspoint; public class Test { public static void main(String[] args) { try { // create an object of the string String str = new String("Welcome to Tutorials Point"); System.out.println("The string value is: " + str); //initialize the index value int index = 50; System.out.println("The index value is: " + index); // use charAt() method System.out.println("The character value at the specifed index " + index + " is: " + str.charAt(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果:
The string value is: Welcome to Tutorials Point The index value is: 50 java.lang.StringIndexOutOfBoundsException: String index out of range: 50 at java.base/java.lang.StringLatin1.charAt(StringLatin1.java:48) at java.base/java.lang.String.charAt(String.java:1515) at com.tutorialspoint.Test.main(Test.java:13) Exception: java.lang.StringIndexOutOfBoundsException: String index out of range: 50
使用 For 循环检索字符串的所有字符示例
使用for 循环以及charAt() 方法,我们可以检索指定索引处的全部字符。
在此程序中,我们使用值为"TutorialsPoint"实例化字符串类。然后,循环将遍历给定的序列。使用charAt() 方法,我们尝试检索指定索引处的全部字符。
package com.tutorialspoint; public class ClassDemo { public static void main(String[] args) { // create an object of the String String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); System.out.print("The characters of the given string are: "); // using for loop along with the charAt() method for (int i = 0; i < str.length(); i++) { System.out.print(str.charAt(i) + " "); } } }
输出
The given string is: TutorialsPoint The characters of the given string are: T u t o r i a l s P o i n t