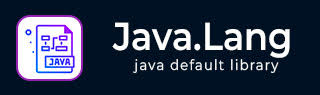
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String codePointAt() 方法
描述
Java String codePointAt() 方法用于检索指定索引处的字符(Unicode 代码点)。索引指的是给定字符串的 char 值的位置,范围从 0 到 length()–1。
第一个字符值表示 第 0 个索引,第二个字符值表示 第 1 个索引 值,以此类推。codePointAt() 方法接受一个整数参数,该参数保存索引值。如果索引值为负数或大于字符串长度,则会抛出异常。
语法
以下是 Java String codePointAt() 方法的语法:
public int codePointAt(int index)
参数
- index − 这是 char 值的索引。
返回值
此方法返回索引处字符的代码点值。
检查特定索引处字符的代码点值示例
如果索引值大于 0 且小于字符串长度,则此方法返回指定索引处字符的代码点。
在下面的示例中,我们使用值为 "JavaProgramming" 创建一个 string 类 的对象。然后,使用 codePointAt() 方法,我们尝试检索索引 3 处的字符代码点值。
package com.tutorialspoint.String; public class Demo { public static void main(String[] args) { //create an object of the string String str = new String("JavaProgramming"); System.out.println("The given string is: " + str); // initialize the index value int index = 3; System.out.println("The given index value is: " + index); //using the codePointAt() method System.out.println("The character code point value of the " + index + "rd index is: " + str.codePointAt(index)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: JavaProgramming The given index value is: 3 The character code point value of the 3rd index is: 97
在检查无效索引处字符的代码点值时出现异常示例
如果索引值大于字符串长度,则此方法将抛出 StringIndexOutOfBoundsException。
在此示例中,我们使用值为 "HelloWorld" 创建一个字符串字面量,然后我们使用 codePointAt() 方法打印指定索引处的字符代码点值,该值的索引大于字符串长度。
package com.tutorialspoint.String; public class Test { public static void main(String[] args) { try { // string initialization String str = "HelloWorld"; System.out.println("The given string is: " + str); //initialize the index value int index = 15; System.out.println("The given index value is: " + index); //using the codePointAt() method System.out.print("The character code point value at the " + index + "th index is: " + str.codePointAt(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given string is: HelloWorld The given index value is: 15 java.lang.StringIndexOutOfBoundsException: index 15, length 10 at java.base/java.lang.String.checkIndex(String.java:4563) at java.base/java.lang.String.codePointAt(String.java:1545) at com.tutorialspoint.String.Test.main(Test.java:13) Exception: java.lang.StringIndexOutOfBoundsException: index 15, length 10
使用给定索引处字符的代码点值执行 For 循环示例
使用 for 循环以及 codePointAt() 方法,我们可以打印给定字符串的所有字符代码点值。
在下面的示例中,我们使用值为 "HelloWorld" 实例化 string 类。然后,使用 codePointAt() 方法以及 for 循环来打印给定字符串的所有字符代码点值。
package com.tutorialspoint.StringBuilder; public class TP { public static void main(String[] args) { // decalre a string String string; // initialize it string = "TutorialsPoint"; System.out.print("The string value is: " + string); // declare integer variables System.out.print("\nThe uni code point of the characters are: "); for (int i = 0; i < string.length(); i++) { System.out.print(string.codePointAt(i) + ","); } } }
输出
上述程序产生以下结果:
The string value is: TutorialsPoint The uni code point of the characters are: 84,117,116,111,114,105,97,108,115,80,111,105,110,116,
在检查负索引处字符的代码点值时出现异常示例
如果给定的索引值为负值,则 codePointAt() 方法将抛出 StringIndexOutOfBoundsException。
package com.tutorialspoint.String; public class Test { public static void main(String[] args) { try { // create an object of the string String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); //initialize the index value int index = -1; System.out.println("The given index value is: " + index); //using the codePointAt() method System.out.print("The character code point value at the " + index + "the index is: " + str.codePointAt(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
执行上述程序后,将产生以下输出:
The given string is: TutorialsPoint The given index value is: -1 java.lang.StringIndexOutOfBoundsException: index -1, length 14 at java.base/java.lang.String.checkIndex(String.java:4563) at java.base/java.lang.String.codePointAt(String.java:1545) at com.tutorialspoint.String.Test.main(Test.java:13) Exception: java.lang.StringIndexOutOfBoundsException: index -1, length 14