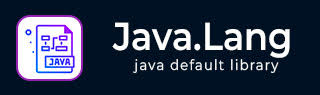
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String codePointBefore() 方法
描述
Java String codePointBefore() 方法返回指定索引之前字符的代码点值(Unicode 代码点)。索引指的是给定字符串中字符值的位置(Unicode 代码单元),范围从1 到长度。
索引值从1 开始,到字符串长度结束,因为如果我们将索引值从0开始,它将开始搜索零索引值之前的字符值,即-1。在这种情况下,codePointBefore() 方法将抛出异常。
codePointBefore() 方法接受一个整数参数,该参数保存索引值,当索引值小于 1 或大于字符串长度时,它会抛出异常。
语法
以下是Java String codePointBefore() 方法的语法:
public int codePointBefore(int index)
参数
- index - 这是应该返回的代码点之后的索引。
返回值
此方法返回给定索引之前的 Unicode 代码点值。
在字符串中检查 0 索引之前的代码点时获取异常示例
如果给定的索引值为零,则 codePointBefore() 方法会抛出StringIndexOutOfBoundsException。
在以下示例中,我们使用值"HelloWorld"实例化字符串类。然后,使用codePointBefore()方法,我们尝试检索指定索引 0之前的字符代码点值。
package com.tutorialspoint.String; public class Demo { public static void main(String[] args) { try { //creating an object of the string class String str = new String("HelloWorld"); System.out.println("The string value is: " + str); //initialize the index value int index = 0; System.out.println("The given index value is: " + index); //using the codePointBefore() method System.out.println("The character code point value before specoied index " + index + " is: " + str.codePointBefore(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
执行上述程序后,将产生以下结果:
The string value is: HelloWorld The given index value is: 0 java.lang.StringIndexOutOfBoundsException: String index out of range: 0 at java.base/java.lang.String.codePointBefore(String.java:1578) at com.tutorialspoint.String.Demo.main(Demo.java:13) Exception: java.lang.StringIndexOutOfBoundsException: String index out of range: 0
在字符串中检查 -1 索引之前的代码点时获取异常示例
如果给定的索引值为负数,则此方法会抛出StringIndexOutOfBoundsException。
在此示例中,我们使用值"TutorialsPoint"创建一个字符串字面量。然后,使用codePointBefore()方法,我们尝试检索指定索引 -1之前的字符代码点值。
package com.tutorialspoint.String; public class Test { public static void main(String[] args) { try { // Initialized the string literal String str = "TutorialsPoint"; System.out.println("The string value is: " + str); //initialize the index value int index = -1; System.out.println("The given index values is: " + index); //using the codePointBefore() method System.out.println("The character code point value before the specifed index " + index + " is: " + str.codePointBefore(index)); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The string value is: TutorialsPoint The given index values is: -1 java.lang.StringIndexOutOfBoundsException: String index out of range: -1 at java.base/java.lang.String.codePointBefore(String.java:1578) at com.tutorialspoint.String.Test.main(Test.java:13) Exception: java.lang.StringIndexOutOfBoundsException: String index out of range: -1
在字符串中检查有效索引之前的代码点示例
如果给定的索引值为正数且小于字符串长度,则 codePointBefore() 方法返回指定索引之前的字符代码点。
在以下程序中,我们使用值"Tutorix"创建 String 类的对象。然后,使用codePointBefore()方法,我们尝试检索指定索引 2 和 4之前的字符代码点值。
package com.tutorialspoint.String; import java.lang.*; public class Demo { public static void main(String[] args) { // create an object of the string String str = new String("Tutorix"); System.out.println("The string value is: " + str); //initialize the index values int index1 = 2; int index2 = 4; System.out.println("The given index values are: " + index1 + " and " + index2); //using the codePointBefore() method System.out.println("The character code point value before the specified index " + index1 + " is: " + str.codePointBefore(index1)); System.out.println("The character code point value before the specified index " + index2 + " is: " + str.codePointBefore(index2)); } }
输出
上述程序产生以下结果:
The string value is: Tutorix The given index values are: 2 and 4 The character code point value before the specified index 2 is: 117 The character code point value before the specified index 4 is: 111