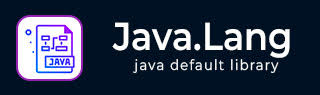
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String compareTo() 方法
描述
Java String compareTo() 方法用于按字典序比较两个字符串。它返回一个整数值,比较基于字符串中每个字符的 Unicode 值。此 String 对象表示的字符序列与参数字符串表示的字符序列按字典序进行比较。
如果此 String 对象按字典序在参数字符串之前,则结果为负整数。
如果此 String 对象按字典序在参数字符串之后,则结果为正整数。
如果字符串相等,则结果为零,当 equals(Object) 方法返回 true 时,compareTo 正好返回 0。
compareTo() 方法接受一个字符串作为参数,并按字典序比较字符串,字典序压缩意味着按字母顺序 (a-z)。在比较给定字符串时,它不会抛出任何异常。
语法
以下是Java String compareTo() 方法的语法 -
public int compareTo(String anotherString)
参数
anotherString - 这是要比较的字符串。
返回值
如果参数字符串等于此字符串,则此方法返回 0。
如果此字符串按字典序小于字符串参数,则此方法返回负值。
如果此字符串按字典序大于字符串参数,则此方法返回正值。
比较与其他字符串相同的字符串示例
如果给定的字符串值相同,则 compareTo() 方法返回零。
在下面的程序中,我们同时使用"Hello" 和"Hello" 两个值实例化两个字符串类。然后,使用compareTo() 方法,我们尝试检查给定的字符串是否按字典序相等。由于给定的字符串按字典序等于字符串参数,因此该方法返回 0。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { //instantiate the string class String str1 = new String("Hello"); String str2 = new String("Hello"); System.out.println("The given strings are: " + str1 + " and " + str2); //using the compare two method System.out.println("The string " + str1 + " is lexicographically equal to the string " + str2 + " or not? " + str1.compareTo(str2)); } }
输出
执行上述程序后,将产生以下结果 -
The given strings are: Hello and Hello The string Hello is lexicographically equal to the string Hello or not? 0
比较按字典序大于其他字符串的字符串示例
如果字符串按字典序大于字符串参数,则此方法返回正值。
在此示例中,我们同时创建了两个字符串字面量,其值为"Tutorials" 和"Point"。使用toCompare() 方法,我们尝试检查给定的字符串是否按字典序相等。由于给定的字符串按字典序大于字符串参数,因此该方法返回正值。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { //instantiate the string class String str1 = "Tutorials"; String str2 = "Point"; System.out.println("The given strings are: " + str1 + " and " + str2); //using the compare two method int compare = str1.compareTo(str2); System.out.println("The compareTo() method returns: " + compare); if (compare == 0) { System.out.println("The strings are lexicographically equal"); } else if (compare > 0) { System.out.println("The first string is larger than the second string lexicographically."); } else { System.out.println("The first string is smaller than the second string lexicographically."); } } }
输出
以下是上述程序的输出 -
The given strings are: Tutorials and Point The compareTo() method returns: 4 The first string is larger than the second string lexicographically.
比较按字典序小于其他字符串的字符串示例
如果字符串等于字符串参数,但参数字符串大小写不同,则 compareTo() 方法返回正值。
在以下示例中,我们使用值 "hello" 和 "HELLO" 实例化字符串类。使用 compareTo() 方法,我们尝试按字典序比较字符串。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { // instantiating the String class String str1 = new String("hello");// string String str2 = new String("HELLO");// string argument System.out.println("The given strings are: " + str1 + " and " + str2); //using the compareTo() method int val = str1.compareTo(str2); System.out.println("The str1.compareTo(str2) value is: " + val); if(val == 0) { System.out.println("The string " + str1 + " and string argument " + str2 + " are lexicographically equal"); } else if(val > 0) { System.out.println("The string " + str1 + " is lexicographically greater than the string argument " + str2); } else { System.out.println("The string " + str1 + " is lexicographically less than the string argument " + str2); } } }
输出
执行程序后,将返回以下输出 -
The given strings are: hello and HELLO The str1.compareTo(str2) value is: 32 The string hello is lexicographically greater than the string argument HELLO