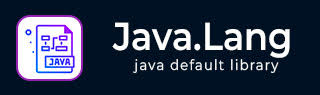
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String compareToIgnoreCase() 方法
描述
Java String compareToIgnoreCase() 方法用于按字典顺序比较两个字符串,忽略大小写差异。字典顺序比较意味着比较可以按字母顺序(a-z)进行。
compareToIgnoreCase() 方法接受一个字符串参数,该参数保存字符串参数的值。如果字符串在字典上相等,则返回一个整数值零;如果当前字符串在字典上大于字符串参数,则返回正值;否则返回负值。
注意 - compareToIgnoreCase() 方法不区分大小写,因为它忽略字符的大小写。
语法
以下是Java String compareToIgnoreCase() 方法的语法:
public int compareToIgnoreCase(String str)
参数
str − 这是要比较的字符串。
返回值
此方法返回一个负整数、零或正整数,具体取决于指定的字符串大于、等于或小于此字符串(忽略大小写)。
忽略大小写比较两个相等字符串的示例
如果当前字符串和给定字符串的值相同,则 compareToIgnoreCase() 方法返回零 (0)。
在下面的程序中,我们用值“Hello”和“Hello”实例化字符串类。使用compareToIgnoreCase() 方法,我们尝试比较字符串是否在字典上相等。由于字符串在字典上相等,因此它返回0。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { //Instantiate the string class String str1 = new String("Hello"); String str2 = new String("Hello"); System.out.println("The given string values are: " + str1 + " and " + str2); // using the compareToIgnoreCase() method int compare = str1.compareToIgnoreCase(str2); System.out.println("The given strings are lexicographically equal. " + compare); } }
输出
执行上述程序后,将产生以下结果:
The given string values are: Hello and Hello The given strings are lexicographically equal. 0
忽略大小写比较两个不相等字符串的示例
如果给定的字符串值与字符串参数不同,则此方法返回一个负值。
在下面的示例中,我们使用值“Java”和“Programming”创建一个字符串类的对象。使用compareToIgnoreCase() 方法,我们尝试按字典顺序比较字符串。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { //creating an object of the string class String str1 = new String("Java"); String str2 = new String("Programming"); System.out.println("The given string values are: " + str1 + " and " + str2); // using the compareToIgnoreCase() method int compare = str1.compareToIgnoreCase(str2); System.out.println("The compareToIgnoreCase() method returns: " + compare); System.out.println("The given strings are not lexicographically equal."); } }
输出
以下是上述程序的输出:
The given string values are: Java and Programming The compareToIgnoreCase() method returns: -6 The given strings are not lexicographically equal
忽略大小写比较两个相等字符串的示例
如果给定的字符串值相同但大小写不同,则 compareToIgnoreCase() 返回零。
在下面的程序中,我们创建了值为“Java”和“JAVA”的字符串文字。使用 compareToIgnoreCase() 方法,我们尝试按字典顺序比较字符串。由于该方法忽略大小写,因此它返回零。
package com.tutorialspoint; public class Compare { public static void main(String[] args) { //creating an object of the string class String str1 = new String("Java"); String str2 = new String("JAVA"); System.out.println("The given string values are: " + str1 + " and " + str2); // using the compareToIgnoreCase() method int compare = str1.compareToIgnoreCase(str2); System.out.println("The compareToIgnoreCase() method returns: " + compare); if(compare == 0) { System.out.println("The given strings are lexicographically equal."); } else if(compare > 0) { System.out.println("The given string is lexicographically greater than the string argument."); } else { System.out.println("The given string is lexicographically less than the string argument."); } } }
输出
程序执行后,将返回以下输出:
The given string values are: Java and JAVA The compareToIgnoreCase() method returns: 0 The given strings are lexicographically equal.