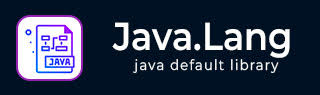
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包扩展
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String concat() 方法
描述
Java String concat() 方法用于将指定的字符串连接到此字符串的末尾。连接是将两个或多个字符串组合成一个新字符串的过程。由于 Java 中的字符串是不可变的,因此一旦初始化,我们就无法更改字符串值。
String 是一个保存字符序列的对象。concat() 方法接受一个字符串作为参数,该字符串保存另一个字符串的值。如果一个字符串的值为 null,则会抛出异常。
语法
以下是 Java String concat() 方法的语法:
public String concat(String str)
参数
- str - 这是连接到此字符串末尾的字符串。
返回值
此方法返回一个字符串值,该值是当前字符串和给定值连接操作的结果。
连接两个非空字符串示例
如果给定的字符串值不为 null,则 concat() 方法会将它们连接起来并返回一个新的字符串。
在下面的程序中,我们使用值“Hello”和“World”创建了一个字符串类的对象。使用concat() 方法,我们尝试将它们连接起来。
package com.tutorialspoint; public class Concat { public static void main(String[] args) { //create an object of the String String str1 = new String("Hello"); String str2 = new String("World"); System.out.println("The given string values are: " + str1 + " and " + str2); //using the concat() method System.out.println("After concatenation the new string is: " + str1.concat(str2)); } }
输出
执行上述程序后,将产生以下结果:
The given string values are: Hello and World After concatenation the new string is: HelloWorld
连接空字符串时出现异常的示例
如果给定的字符串参数值为null,则此方法会抛出NullPointerException。
在下面的示例中,我们使用值“Tutorials”实例化 String 类。使用concat() 方法,我们尝试将当前字符串值与null连接起来。
public class Concat { public static void main(String[] args) { try { //instantiate the String class String str1 = new String("Hello"); String str2 = new String(); str2 = null; System.out.println("The given string values are: " + str1 + " and " + str2); //using the concat() method System.out.println("After concatenation the new string is: " + str1.concat(str2)); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given string values are: Hello and null java.lang.NullPointerException: Cannot invoke "String.isEmpty()" because "str" is null at java.base/java.lang.String.concat(String.java:2769) at com.tutorialspoint.StringBuilder.Concat.main(Concat.java:11) Exception: java.lang.NullPointerException: Cannot invoke "String.isEmpty()" because "str" is null
连接两个空字符串的示例
如果给定的字符串值为空,则 concat() 方法将返回一个空字符串。
在此程序中,我们使用空值创建字符串字面量。使用concat() 方法,我们将空字符串值连接起来。由于这些值为空,因此此方法返回一个空字符串。
package com.tutorialspoint; public class Concat { public static void main(String[] args) { try { //create the String literals String str1 = ""; String str2 = ""; System.out.println("The given string values are: " + str1 + ", " + str2); //using the concat() method System.out.println("After concatenation the new string is: " + str1.concat(str2)); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
执行程序后,将返回以下输出:
The given string values are: , After concatenation the new string is:
java_lang_string.htm
广告