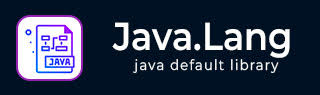
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String contains() 方法
描述
Java String contains() 方法用于检查当前字符串是否包含指定的序列。它返回布尔值,当且仅当字符串包含字符值的序列时返回 true;否则返回 false。
contains() 方法接受一个字符序列作为参数。如果序列之一包含空值,则会抛出异常。字符序列是字符值的可读序列。
语法
以下是Java String contains() 方法的语法:
public boolean contains(CharSequence s)
参数
s - 这是要搜索的序列。
返回值
如果此字符串包含 s,则此方法返回 true,否则返回 false。
检查字符串是否包含 CharSequence 示例
如果给定的字符串值包含指定的字符序列值,则 contains() 方法返回true。
在下面的程序中,我们使用值“Java Programming”实例化字符串类。然后,我们使用值“Java”创建字符序列。使用contains() 方法,我们尝试检查给定字符串是否包含指定的字符序列。
package com.tutorialspoint; public class Concat { public static void main(String[] args) { //instantiate the string class String str1 = new String("Java Programming"); System.out.println("The given string: " + str1); //initialize the char sequence CharSequence str2 = "Java"; System.out.println("The given char sequence is: " + str2); //using the contains() method boolean bool = str1.contains(str2); System.out.println("The string contains the specified char sequence or not? " + bool); } }
输出
执行上述程序后,将产生以下结果:
The given string: Java Programming The given char sequence is: Java The string contains the specified char sequence or not? true
检查字符串是否不包含 CharSequence 示例
如果给定的字符串值不包含指定的字符序列值,则 contains() 方法返回false。
在下面的程序中,我们使用值“HelloWorld”创建一个字符串类对象。然后,我们使用值“helo”创建字符序列。使用contains() 方法,我们尝试检查给定字符串是否包含指定的字符序列。
package com.tutorialspoint; public class Concat { public static void main(String[] args) { // creating an object the string class String str1 = new String("HelloWorld"); System.out.println("The given string: " + str1); //initialize the char sequence CharSequence str2 = "helo"; System.out.println("The given char sequence is: " + str2); //using the contains() method boolean bool = str1.contains(str2); System.out.println("The string contains the specified char sequence or not? " + bool); } }
输出
以下是上述程序的输出:
The given string: HelloWorld The given char sequence is: helo The string contains the specified char sequence or not? false
在检查字符串是否包含 CharSequence 时获取异常的示例
如果给定的字符序列值为null,则此方法会抛出NullPointerException。
在此程序中,我们使用值“hello”创建一个字符串字面量。然后,我们使用null 值创建一个字符序列。使用contains() 方法,我们尝试检查给定字符串是否包含指定的字符序列 null。
public class Concat { public static void main(String[] args) { try { // instantiate the string class String str1 = "hello"; System.out.println("The given string: " + str1); CharSequence str2 = null; System.out.println("The given char sequence is: " + str2); //using the contains() method boolean bool = str1.contains(str2); System.out.println("The string conatins the specified char seqience or not? " + bool); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下输出:
The given string: hello The given char sequence is: null java.lang.NullPointerException: Cannot invoke "java.lang.CharSequence.toString()" because "s" is null at java.base/java.lang.String.contains(String.java:2854) at com.tutorialspoint.StringBuilder.Concat.main(Concat.java:11) Exception: java.lang.NullPointerException: Cannot invoke "java.lang.CharSequence.toString()" because "s" is null
在区分大小写模式下检查字符串是否包含 CharSequence 的示例
如果给定的字符串和字符序列值相同,但大小写不同,则 contains() 方法返回false。
在此示例中,我们使用值“TUTORIX”实例化字符串类。然后,我们使用值“tutorix”创建字符序列。使用contains() 方法,我们尝试比较字符串是否包含指定的字符序列。
package com.tutorialspoint; public class Concat { public static void main(String[] args) { // instantiate the string class String str1 = new String("TUTORIX"); System.out.println("The given string: " + str1); CharSequence str2 = "tutorix"; System.out.println("The given char sequence is: " + str2); //using the contains() method boolean bool = str1.contains(str2); System.out.println("The contains() method return: " + bool); if(bool) { System.out.println("The given string contains the spcified char sequence"); } else { System.out.println("The given string does not contain the specified char sequence"); } } }
输出
执行上述程序后,将生成以下输出:
The given string: TUTORIX The given char sequence is: tutorix The contains () method return: false The given string does not contain the specified char sequence