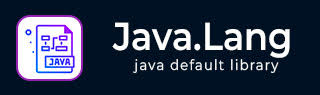
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包补充
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang -有用资源
- Java.lang - 讨论
Java - String contentEquals() 方法
描述
Java String contentEquals() 方法用于比较当前字符串与给定的字符序列。此方法返回一个布尔值,当且仅当此字符串表示与指定序列相同的 char 值序列时返回 true;否则返回 false。
contentEquals() 方法接受两个参数,分别是 CharSequence 和 StringBuffer,它们分别保存 cs 和 sb 值。在将当前字符串与指定的字符序列和字符串缓冲区进行比较时,它不会抛出任何异常。
CharSequence 是字符值的易读序列。字符串是字符的不可变序列,并实现 CharSequence 接口。StringBuffer 与字符串类似,但它是可修改的且同步的。
contentEquals() 方法有两个多态变体,它们具有不同的参数:CharSequence 和 StringBuffer(以下是所有多态变体的语法)。
语法
以下是Java String contentEquals() 方法的语法:
public boolean contentEquals(CharSequence cs) public Boolean contentEquals(StringBuffer sb)
参数
cs − 这是要与该字符串进行比较的字符序列。
sb − 这是要与该字符串进行比较的 StringBuffer 序列。
返回值
此方法返回一个布尔值,如果当前字符串与给定对象相等则为 True,否则为 false。
使用 CharSequence 示例检查匹配字符串
如果我们将CharSequence作为参数传递给此方法,并且它与当前字符串具有相同的值,则 contentEquals() 方法将返回true。
在下面的程序中,我们使用值为"Hello"创建了一个字符串字面量。然后,我们使用相同的值"Hello"创建了一个 CharSequence。由于两者具有相同的值,因此当我们使用contentEquals()方法比较它们时,此方法将返回 true。
package com.tutorialspoint; public class TP { public static void main(String[] args) { // create a string literal String str1 = "Hello"; System.out.println("The String is: " + str1); //create a charSequence CharSequence ch = "Hello"; System.out.println("The given char sequence is: " + ch); System.out.println("The given string is equal to the specified sequence or not? " + str1.contentEquals(ch)); } }
输出
执行上述程序后,将产生以下结果:
The String is: Hello The given char sequence is: Hello The given string is equal to the specified sequence or not? true
使用 CharSequence 示例检查不匹配字符串
如果我们将 CharSequence 作为参数传递给方法,并且它与当前字符串的值不同,则此方法将返回false。
在下面的程序中,我们使用值为"TutorialsPoint"创建了一个字符串类的对象。然后,使用contentEquals()方法,我们尝试比较指定字符序列处的字符串。由于两者具有不同的值,因此该方法将返回false。
package com.tutorialspoint; public class TP { public static void main(String[] args) { // create an object of the String class String str = new String("TutorialsPoint"); // CharSequance declaration CharSequence cs = "Point"; System.out.println("The string is: " + str); System.out.println("The CharSequence is: " + cs); // declare boolean variable to hold the method value boolean bool = str.contentEquals(cs); System.out.println("The given string is equal to the specified sequence or not? " + bool); } }
输出
以下是上述程序的输出:
The string is: TutorialsPoint The CharSequence is: Point The given string is equal to the specified sequence or not? false
使用 StringBuffer 示例检查匹配字符串
如果我们将StringBuffer作为参数传递给方法,并且它与字符串具有相同的值,则 contentEquals() 方法将返回true。
在下面的程序中,我们使用值为"Hello World"实例化字符串类。然后,我们使用值为"Hello World"创建了一个 StringBuffer 类的对象。使用contentEquals()方法,我们尝试比较指定 StringBuffer 处的字符串。由于两者具有相同的值,因此 contentEquals() 方法将返回true。
package com.tutorialspoint; public class TP { public static void main(String[] args) { // Instantiate the String class String str = new String("Hello World"); // create an object of the StringBuffer class StringBuffer sb = new StringBuffer("Hello World"); System.out.println("The string is: " + str); System.out.println("The StringBuffer is: " + sb); // using contentEquals() method System.out.println("The given string is equal to the specified StringBuffer sequence or not? " + str.contentEquals(sb)); } }
输出
上述程序产生以下输出:
The string is: Hello World The StringBuffer is: Hello World The given string is equal to the specified StringBuffer sequence or not? true
使用 CharSequence 示例检查不匹配字符串
在此程序中,我们使用值为"JavaScript"创建了一个字符串字面量。然后,我们使用值为"Java"创建了一个 StringBuffer。使用contentEquals()方法,我们尝试将字符串与指定的 StringBuffer 进行比较。
package com.tutorialspoint; public class TP { public static void main(String[] args) { // create a string literal String str ="JavaScript"; // Instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Java"); System.out.println("The string is: " + str); System.out.println("The StringBuffer is: " + sb); // using contentEquals() method boolean bool = str.contentEquals(sb); if(bool) { System.out.println("The given string is equal to the specified StringBuffer sequence"); } else { System.out.println("The given string is not equal to the specified StringBuffer sequence"); } } }
输出
执行上述程序后,将生成以下输出:
The string is: JavaScript The StringBuffer is: Java The given string is not equal to the specified StringBuffer sequence
使用 contentEquals() 方法检查字符串时出现异常的示例
如果给定的字符串值为null,则此方法将抛出NullPointerException。
在下面的示例中,我们使用null 值创建了一个字符串。然后,我们使用值为"Java"实例化 StringBuffer 类。使用contentEquals()方法,我们尝试将给定的字符串与指定的 StringBuffer 进行比较。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { try { // create a string literal String str = null; // Instantiate the StringBuffer class StringBuffer sb = new StringBuffer("Java"); System.out.println("The string is: " + str); System.out.println("The StringBuffer is: " + sb); // using contentEquals() method boolean bool = str.contentEquals(sb); if(bool) { System.out.println("The given string is equal to the specified StringBuffer sequence"); } else { System.out.println("The given string is not equal to the specified StringBuffer sequence"); } } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exceptipn: " + e); } } }
输出
以下是上述程序的输出:
The string is: null java.lang.NullPointerException: Cannot invoke "String.contentEquals(StringBuffer)" because "str" is null The StringBuffer is: Java at com.tutorialspoint.String.Demo.main(Demo.java:13) Exceptipn: java.lang.NullPointerException: Cannot invoke "String.contentEquals(StringBuffer)" because "str" is null