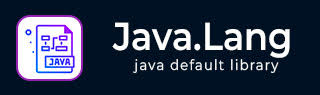
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包补充
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String copyValueOf() 方法
描述
Java String copyValueOf() 方法返回一个表示指定数组中字符序列的字符串。它创建一个新数组并将字符插入其中。
copyValueOf() 方法是一个静态方法,这意味着可以直接使用类名本身访问它。
此方法具有两个多态变体,具有不同的参数:int, char [ ]。(以下是所有多态变体的语法)。
语法
以下是Java String copyValueOf() 方法的语法:
public static String copyValueOf(char[] data)// first syntax public static String copyValueOf(char[] data, int offset, int count)// second syntax
参数
data − 这是字符数组。
offset − 子数组的初始偏移量。
count − 子数组的长度。
返回值
此方法返回一个包含字符数组中字符的字符串。
将字符数组复制到字符串示例
如果数组的字符不为空,则 copyValueOf() 方法返回一个表示 char 数组中字符的字符串。
在下面的程序中,我们创建一个值为'j','a','v' 和 'a' 的 char 数组。使用copyValueOf() 方法,我们尝试检索表示 char 数组中字符的字符串。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { // create an character array char ch[] = {'j','a','v','a'}; System.out.print("The char array elements are: "); for(int i = 0; i<ch.length; i++) { System.out.print(ch[i] + " "); } // using copyValueOf() method String str = String.copyValueOf(ch); // prints the string System.out.println("\nThe string represents the characters of the char array is: " + str); } }
输出
执行上述程序后,将产生以下结果:
The char array elements are: j a v a The string represents the characters of the char array is: java
使用空字符数组时 copyValueOf() 方法引发异常示例
如果给定的字符数组为null,此方法将抛出NullPointerException。
在下面的示例中,我们创建一个值为null 的 char 数组。使用copyValueOf() 方法,我们尝试检索表示给定 char 数组中字符的字符串。由于 char 数组为空,因此会抛出异常。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { try { // create an character array char ch[] = null; System.out.print("The char array elements are: "); for(int i = 0; i<ch.length; i++) { System.out.print(ch[i] + " "); } // using copyValueOf() method String str = String.copyValueOf(ch); // prints the string System.out.println("The string represents the charcaters of the char array is: " + str); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出:
The char array elements are: java.lang.NullPointerException: Cannot read the array length because "ch" is null at com.tutorialspoint.Demo.main(Demo.java:9) Exception: java.lang.NullPointerException: Cannot read the array length because "ch" is null
使用偏移量使用 copyValueOf() 方法复制字符数组示例
如果给定的 char 数组不为空,并且偏移量和计数参数值已传递给方法,则 copyValueOf() 方法将返回表示 char 数组中字符的字符串。
在此程序中,我们创建一个值为'H','E','L','L' 和 'O' 的 char 数组。使用copyValueOf() 方法,我们尝试检索表示 char 数组中字符的字符串,其中偏移量值为1,计数值为4。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { try { // create an character array char ch[] = { 'H', 'E', 'L', 'L', 'O'}; System.out.print("The char array elements are: "); for(int i = 0; i<ch.length; i++) { System.out.print(ch[i] + " "); } //initialize the offset and count value int offset = 1, count = 4; System.out.println("\nThe offset and count values are: " + offset + " and " + count); // using copyValueOf() method String str = String.copyValueOf(ch, offset, count); // prints the string System.out.println("The string represents the characters of the char array is: " + str); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下输出:
The char array elements are: H E L L O The offset and count values are: 1 and 4 The string represents the characters of the char array is: ELLO
使用无效偏移量时 copyValueOf() 方法引发异常示例
如果给定的偏移量值为负数,则 copyValueOf() 方法将抛出StringIndexOutOfBoundsException。
在下面的程序中,我们创建一个值为'H','E','L','L' 和 'O' 的 char 数组。使用copyValueOf() 方法,我们尝试检索表示 char 数组中字符的字符串,其中偏移量值为-1,计数值为2。由于偏移量值为负数,因此会抛出异常。
package com.tutorialspoint; public class Demo { public static void main(String[] args) { try { // create an character array char ch[] = { 'H', 'E', 'L', 'L', 'O'}; System.out.print("The char array elements are: "); for(int i = 0; i<ch.length; i++) { System.out.print(ch[i] + " "); } //initialize the offset and count value int offset = -1, count = 2; System.out.println("\nThe offset and count values are: " + offset + " and " + count); // using copyValueOf() method String str = String.copyValueOf(ch, offset, count); // prints the string System.out.println("The specified returned string is: " + str); } catch(IndexOutOfBoundsException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
执行上述程序后,将生成以下输出:
The char array elements are: H E L L O The offset and count values are: -1 and 2 java.lang.StringIndexOutOfBoundsException: offset -1, count 2, length 5 at java.base/java.lang.String.checkBoundsOffCount(String.java:4589) at java.base/java.lang.String.rangeCheck(String.java:304) at java.base/java.lang.String.<init>(String.java:300) at java.base/java.lang.String.copyValueOf(String.java:4273) at com.tutorialspoint.String.Demo.main(Demo.java:17) Exception: java.lang.StringIndexOutOfBoundsException: offset -1, count 2, length 5