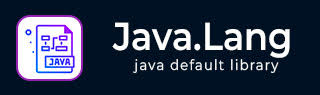
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - String format() 方法
描述
Java String format() 方法用于使用指定的区域设置、格式字符串和对象参数获取格式化的字符串。如果在 String.format() 方法中未指定区域设置,则通过调用 Locale.getDefault() 方法本身使用默认区域设置。
在 Java 中,区域设置是一个表示特定地理、政治或文化区域的对象。format() 方法接受不同的参数,例如区域设置、格式和对象,这些参数分别保存区域设置 l、字符串格式和对象参数的值。
format() 方法有两个多态变体,具有不同的参数,如下面的语法所示。
语法
以下是 Java String format() 方法的语法 -
public static String format(Locale l, String format, Object... args) //first syntax public static String format(String format, Object... args) // second syntax
参数
l - 这是在格式化期间要应用的区域设置。如果为 null,则不应用任何本地化。
format - 这是一个格式字符串。
args - 这是格式字符串中格式说明符引用的参数。如果参数多于格式说明符,则会忽略多余的参数。参数的数量是可变的,可以为零。
返回值
此方法使用指定的区域设置、格式字符串和参数返回格式化的字符串。
使用区域设置获取格式化字符串示例
如果我们将 区域设置、格式和对象参数作为参数传递给此方法,它将返回一个格式化的字符串。
在下面的程序中,我们使用值 "Java Programming" 实例化字符串类。然后,使用 format() 方法,我们尝试在指定的区域设置 "Locale.CANADA" 和对象参数处格式化当前字符串。
package com.tutorialspoint; import java.util.Locale; public class Format { public static void main(String[] args) { //instantiate the string class String str = new String("Ramesh"); System.out.println("The given string is: " + str); System.out.println("After formatting the string.....\n" + String.format(Locale.CANADA, "My name is: %s", str)); } }
输出
执行上述程序后,将产生以下结果 -
The given string is: Ramesh After formatting the string.... My name is: Ramesh
格式化字符串示例
如果我们将 格式和对象参数作为参数传递给方法,则此方法将返回一个格式化的字符串。
在下面的示例中,我们使用值 "TutorialsPoint" 创建字符串类的对象。使用 format() 方法,我们尝试获取当前字符串在指定格式中的格式化字符串。
package com.tutorialspoint; import java.util.Locale; public class Format { public static void main(String[] args) { // create an object of the string class String str = new String("TutorialsPoint"); // initialize the double variable Double d = 234.454d; System.out.println("The given string is: " + str); System.out.println("The given double value is: " + d); // use format() method String new_str; String new_str1; new_str = String.format("Welcome to the %s", str); new_str1 = String.format("The result is: %15f", d); System.out.println("The formatted string is: " + new_str); System.out.println("The formatted value is: " + new_str1); } }
输出
以下是上述程序的输出 -
The given string is: TutorialsPoint The given double value is: 234.454 The formatted string is: Welcome to the TutorialsPoint The formatted value is: The result is: 234.454000
检查格式化字符串时出现的 NullPointerException 示例
如果格式值为 null,则 format() 方法将返回 NullPointerException。
在此示例中,我们使用值 "Hello" 创建字符串类的实例。然后,使用 format() 方法,我们尝试获取当前字符串在指定格式 null 中的格式化字符串。
package com.tutorialspoint; import java.util.Locale; public class Format { public static void main(String[] args) { try { // create a string literal String str1 = new String("Hello"); System.out.println("The given string is: " + str1); // using format() method String new_str = String.format(Locale.CANADA, null, str1); } catch(Exception e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
上述程序产生以下结果 -
The given string is: Hello java.lang.NullPointerException: Cannot invoke "String.length()" because "s" is null at java.base/java.util.Formatter.parse(Formatter.java:2717) at java.base/java.util.Formatter.format(Formatter.java:2671) at java.base/java.util.Formatter.format(Formatter.java:2625) at java.base/java.lang.String.format(String.java:4184) at com.tutorialspoint.String.Format.main(Format.java:11) Exception: java.lang.NullPointerException: Cannot invoke "String.length()" because "s" is null
检查格式化字符串时出现的 IllegalFormatException 示例
如果我们将 非法格式语法传递给方法,它将抛出 IllegalFormatException。
在此程序中,我们使用值 "Tutorix" 创建字符串类的对象。然后,使用 format() 方法,我们尝试获取当前字符串对象在指定格式 "%d" 中的格式化字符串。由于我们对字符串使用 "%d" 格式,因此该方法将抛出异常。
package com.tutorialspoint; import java.util.Locale; public class Format { public static void main(String[] args) { try { // create an object of the string class String str = new String("Tutorix"); System.out.println("The given string is: " + str); // using format method we pass String new_string = String.format("%d", str); System.out.println("The new formatted string is: " + new_string); } catch(Exception e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
执行上述程序后,将产生以下输出 -
The given string is: Tutorix java.util.IllegalFormatConversionException: d != java.lang.String at java.base/java.util.Formatter$FormatSpecifier.failConversion(Formatter.java:4442) at java.base/java.util.Formatter$FormatSpecifier.printInteger(Formatter.java:2963) at java.base/java.util.Formatter$FormatSpecifier.print(Formatter.java:2918) at java.base/java.util.Formatter.format(Formatter.java:2689) at java.base/java.util.Formatter.format(Formatter.java:2625) at java.base/java.lang.String.format(String.java:4143) at com.tutorialspoint.String.Format.main(Format.java:10) Exception: java.util.IllegalFormatConversionException: d != java.lang.String