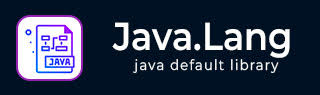
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String getBytes() 方法
描述
Java String getBytes() 方法用于使用平台的默认字符集将此字符串编码(转换)为字节序列,并将结果存储到新的字节数组中。在 Java 中,数组是一个包含相似数据类型元素的对象。字节是内存中最小的可寻址单元。
注意:int 数据类型的尺寸为 4 个字节,char 数据类型的尺寸为 1 个字节,依此类推。
getBytes() 方法接受字符集和字符串作为参数,其中包含字符集和字符集名称的值。如果字符集或字符集名称无效,则会抛出异常。
此方法具有三个具有不同参数的多态变体,如下面的语法所示。
语法
以下是Java String getBytes() 方法的语法:
public byte[] getBytes() // first syntax public byte[] getBytes(Charset charset) // second syntax public byte[] getBytes(String charsetName) // third syntax
参数
- charset − 这是十六位 Unicode 代码单元序列和字节序列之间的命名映射。
- charsetName − 这是字符串。
返回值
此方法使用给定的值将此字符串编码为字节序列,并以字节数组的形式返回结果。
获取字符串的字节示例
如果我们不向方法传递默认字符集,它将通过获取默认字符集本身来将此字符串编码为字节序列。
在下面的程序中,我们使用值“Hello World”实例化字符串类。然后,使用getBytes() 方法,我们尝试将当前字符串转换为字节序列并将它存储在字节数组中。
package com.tutorialspoint; import java.util.Arrays; public class GetByte { public static void main(String[] args) { //instantiate the string class String str = new String("Hello World"); System.out.println("The given string is: " + str); // create a byte array byte[] byte_arr; // using getBytes() method byte_arr = str.getBytes(); // print the byte array value System.out.println("The byte array is: " + Arrays.toString(byte_arr)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Hello World The byte array is: [72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]
使用 UTF-8 编码获取字符串的字节示例
如果我们将charset 传递给 getBytes() 方法,此方法将使用给定的字符集将此字符串编码为字节序列。
在下面的示例中,我们使用值“Java”创建一个字符串类的对象。使用getBytes() 方法,我们尝试使用字符集“UTF-8”将当前字符串编码为字节数组。
package com.tutorialspoint; import java.nio.charset.Charset; import java.util.Arrays; public class GetByte { public static void main(String[] args) { try { //create an object of the string class String str = new String("Java"); System.out.println("The given string is: " + str); // copy the contents of the String to a byte array System.out.println("The charset is: " + Charset.forName("UTF-8")); byte[] arr = str.getBytes(Charset.forName("UTF-8")); System.out.println("The byte array is: "); for(int i = 0; i<arr.length; i++) { System.out.print(arr[i] + " "); } } catch (Exception e) { System.out.print(e.toString()); } } }
输出
以下是上述程序的输出:
The given string is: Java The charset is: UTF-8 The byte array is: 74 97 118 97
使用 UTF-16 编码获取字符串的字节示例
如果我们将字符串charsetName 作为参数传递给该方法,它将使用给定的字符集名称将此字符串编码为字节序列。
在下面的程序中,我们使用值“TutorialsPoint”创建一个字符串字面量。使用getBytes() 方法,我们尝试使用字符集名称“UTF-16”将当前字符串编码为字节数组。
package com.tutorialspoint; import java.nio.charset.Charset; public class GetByte { public static void main(String[] args) { try { // create a string literal String str= "TutoriaslPoint"; System.out.println("The given string is: " + str); System.out.println("The charset name is: " + Charset.forName("UTF-16")); // byte array with charset byte bval[] = str.getBytes("UTF-8"); // prints the byte array System.out.print("The byte array elements are: "); for (int i = 0; i < bval.length; i++) { System.out.print(bval[i] + " "); } } catch (Exception e) { e.printStackTrace(); System.out.println("The exception is: " + e); } } }
输出
上述程序产生以下输出:
The given string is: TutoriaslPoint The charset name is: UTF-16 The byte array elements are: 84 117 116 111 114 105 97 115 108 80 111 105 110 116
使用无效编码获取字符串的字节时出现异常的示例
如果给定的字符集或字符集名称无效,getBytes() 方法将抛出UnsupportedEncodingException。
在下面的程序中,我们使用值“Tutorix”实例化一个字符串类。然后,使用getBytes() 方法,我们尝试使用字符集名称“UTF-50”将当前字符串转换为字节序列。由于字符集名称无效,该方法将抛出异常。
package com.tutorialspoint; import java.io.UnsupportedEncodingException; import java.nio.charset.Charset; import java.util.Arrays; public class GetByte { public static void main(String[] args) { try { //instantiate the string class String str = new String("Tutorix"); System.out.println("The given string is: " + str); System.out.println("The charset name is: " + Charset.forName("UTF-50")); // copy the contents of the String to a byte array byte[] arr = str.getBytes("UTF-50"); System.out.println("The byte array is: " + Arrays.toString(arr)); } catch (UnsupportedEncodingException e) { System.out.print(e.toString()); } } }
输出
执行上述程序后,将产生以下输出:
The given string is: Tutorix Exception in thread "main" java.nio.charset.UnsupportedCharsetException: UTF-50 at java.base/java.nio.charset.Charset.forName(Charset.java:528) at com.tutorialspoint.String.GetByte.main(GetByte.java:12)