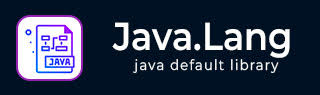
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String getChars() 方法
描述
Java String getChars() 方法用于将此字符串中的字符复制到目标字符数组中。在 Java 中,数组是一个存储相似类型元素的对象。
getChars() 方法接受四个不同的参数,这些参数保存源起始索引、源结束索引、目标字符数组和目标起始索引的值。如果 srcBegin 值为负数,srcBegin 大于 srcEnd,srcEnd 大于字符串等,则会抛出异常。
第一个要复制的字符位于 srcBegin 索引处,最后一个要复制的字符位于 srcEnd-1 索引处。
语法
以下是 Java String getChars() 方法的语法:
public void getChars(int srcBegin, int srcEnd, char[] dst, int dstBegin)
参数
srcBegin - 这是要复制的字符串中第一个字符的索引。
srcEnd - 这是要复制的字符串中最后一个字符之后的索引。
dst - 这是目标数组。
dstBegin - 这是目标数组中的起始偏移量。
返回值
此方法不返回值。
从字符串获取字符数组示例
如果 srcBegin 值为正数,并且 srcEnd 值小于字符串长度,则 getChars() 方法会将字符串字符复制到字符数组中。
在下面的程序中,我们使用“Welcome to TutorialsPoint”的值实例化一个字符串类。使用getChars() 方法,我们尝试将字符串字符复制到字符数组中,指定的srcIndex 为 5,srcEnd 为 15,dstbegin 为 0。
package com.tutorialspoint; import java.util.Arrays; public class GetChars { public static void main(String[] args) { //instantiate the string class String str = new String("Welcome to TutorialsPoint"); System.out.println("The given string is: " + str); // create character array char[] chararr = new char[10]; // copies characters from starting and ending index into the destination character array //initialize the srcBegin, srcEnd, and dstBegin values int srcBegin = 5, srcEnd = 15, dstBegin = 0; System.out.println("The srcBegin, srcEnd, and dstBegin values are: " + srcBegin + ", " + srcEnd + ", and " + dstBegin); //using the getChars() method str.getChars(srcBegin, srcEnd, chararr, dstBegin); // print the character array System.out.print("The Value of character array : " + Arrays.toString(chararr)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Welcome to TutorialsPoint The srcBegin, srcEnd, and dstBegin values are: 5, 15, and 0 The Value of character array : [m, e, , t, o, , T, u, t, o]
从字符串获取字符数组,起始索引为 -1 的示例
如果给定的 srcBegin 索引值为负数,则 getChars() 方法会抛出StringIndexOutOfBoundsException异常。
在以下示例中,我们使用值“Hello World”创建了一个字符串类的对象。然后,我们创建一个大小为 20的字符数组。使用getChars() 方法,我们尝试将字符串字符从当前字符串复制到字符数组中,指定的srBegin 索引为 -1,srcEnd 索引为 5,dstBegin 索引为 0。
package com.tutorialspoint; import java.util.Arrays; public class GetChars { public static void main(String[] args) { try { //create an object of the string class String str = new String("Hello World"); System.out.println("The given string is: " + str); // create character array char[] chararr = new char[20]; // copies characters from starting and ending index into the destination character array //initialize the srcBegin, srcEnd, and dstBegin index values int srcBegin = -1, srcEnd = 5, dstBegin = 0; System.out.println("The srcBegin, secEnd, and dstBegin index value are: " + srcBegin + ", " + srcEnd + ", and " + dstBegin ); //using the getChars() method str.getChars(srcBegin, srcEnd, chararr, dstBegin); // print the character array System.out.print("The Value of character array : " + Arrays.toString(chararr)); } catch (StringIndexOutOfBoundsException e) { e.printStackTrace(); System.out.print("Exception: " + e); } } }
输出
以下是上述程序的输出:
The given string is: Hello World The srcBegin, secEnd, and dstBegin index value are: -1, 5, and 0 java.lang.StringIndexOutOfBoundsException: begin -1, end 5, length 11 at java.base/java.lang.String.checkBoundsBeginEnd(String.java:4604) at java.base/java.lang.String.getChars(String.java:1676) at com.tutorialspoint.String.GetChars.main(GetChars.java:17) Exception: java.lang.StringIndexOutOfBoundsException: begin -1, end 5, length 11
检查从字符串获取字符数组时发生的异常示例
如果 srcBegin 值大于 srcEnd,则此方法会抛出StringIndexOutOfBoundsException异常。
在此示例中,我们使用值“Java Programming”创建一个字符串。使用getChars() 方法,我们尝试将字符串字符从当前字符串复制到字符数组中,指定的srBegin 索引为 5,srcEnd 索引为 3,dstBegin 索引为 1。由于 serBegin 值大于 srEnd,因此该方法将抛出异常。
import java.util.Arrays; public class GetChars { public static void main(String[] args) { try { //create an object of the string class String str = new String("Java Programming"); System.out.println("The given string is: " + str); // create character array char[] chararr = new char[25]; // copies characters from starting and ending index into the destination character array //initialize the srcBegin, srcEnd, and dstBegin index values int srcBegin = 5, srcEnd = 3, dstBegin = 1; System.out.println("The srcBegin, secEnd, and dstBegin index value are: " + srcBegin + ", " + srcEnd + ", and " + dstBegin ); //using the getChars() method str.getChars(srcBegin, srcEnd, chararr, dstBegin); // print the character array System.out.print("The Value of character array : " + Arrays.toString(chararr)); } catch (StringIndexOutOfBoundsException e) { e.printStackTrace(); System.out.print("Exception: " + e); } } }
输出
上述程序产生以下输出:
The given string is: Java Programming The srcBegin, secEnd, and dstBegin index value are: 5, 3, and 1 java.lang.StringIndexOutOfBoundsException: begin 5, end 3, length 16 at java.base/java.lang.String.checkBoundsBeginEnd(String.java:4604) at java.base/java.lang.String.getChars(String.java:1676) at com.tutorialspoint.String.GetChars.main(GetChars.java:17) Exception: java.lang.StringIndexOutOfBoundsException: begin 5, end 3, length 16
检查从字符串获取字符数组时发生的异常示例
如果 srcEnd 值大于字符串长度,则 getChars() 方法会抛出StringIndexOutOfBoundsException异常。
在下面的程序中,我们使用值“TutorialsPoint”实例化字符串类。使用getChars() 方法,我们尝试将字符串字符从此字符串复制到字符数组中,指定的 srBegin 索引为 1,srcEnd 索引为 15,其值大于字符串长度。
import java.util.Arrays; public class GetChars { public static void main(String[] args) { try { //create an object of the string class String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); System.out.println("The string length is: " + str.length()); // create character array char[] chararr = new char[20]; // copies characters from starting and ending index into the destination character array //initialize the srcBegin, srcEnd, and dstBegin index values int srcBegin = 1, srcEnd = 15, dstBegin = 0; System.out.println("The srcBegin, secEnd, and dstBegin index value are: " + srcBegin + ", " + srcEnd + ", and " + dstBegin ); //using the getChars() method str.getChars(srcBegin, srcEnd, chararr, dstBegin); // print the character array System.out.print("The Value of character array : " + Arrays.toString(chararr)); } catch (StringIndexOutOfBoundsException e) { e.printStackTrace(); System.out.print("Exception: " + e); } } }
输出
执行上述程序后,将生成以下输出:
The given string is: TutorialsPoint The string length is: 14 The srcBegin, secEnd, and dstBegin index value are: 1, 15, and 0 java.lang.StringIndexOutOfBoundsException: begin 1, end 15, length 14 at java.base/java.lang.String.checkBoundsBeginEnd(String.java:4604) at java.base/java.lang.String.getChars(String.java:1676) at com.tutorialspoint.String.GetChars.main(GetChars.java:18) Exception: java.lang.StringIndexOutOfBoundsException: begin 1, end 15, length 14