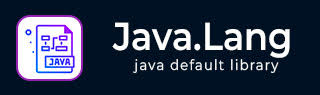
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String intern() 方法
描述
Java String intern() 方法用于检索当前字符串对象的规范表示形式。简而言之,intern() 方法用于在堆内存中创建字符串的精确副本,并将其存储在字符串常量池中。池是 Java 堆内存中一个特殊的存储空间,字符串字面量可以存储在此处。
规范表示形式表示特定类型资源的值可以用多种方式描述或表示,其中一种方式被选为首选的规范形式。
注意 - 可以通过测试其规范形式的相等性来轻松测试两个对象的相等性。
语法
以下是Java String intern() 方法的语法 -
public String intern()
参数
它不接受任何参数。
返回值
此方法返回字符串对象的规范表示形式。
创建当前字符串副本示例
如果给定的字符串不为空,则 intern() 方法会在堆内存中创建当前字符串对象的相同副本。
在下面的程序中,我们使用值“TutorialsPoint”实例化字符串类。使用intern() 方法,我们尝试在堆内存中创建当前字符串的精确副本。
package com.tutorialspoint; public class Intern { public static void main(String[] args) { //instantiate the string class String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); //using the intern() method String str1 = str.intern(); System.out.println("The canonical representation of the current string : " + str1); } }
输出
执行上述程序后,将产生以下结果 -
The given string is: TutorialsPoint The canonical representation of the current string: TutorialsPoint
创建当前字符串副本时出现空指针异常示例
如果给定的字符串具有空值,则 intern() 方法会抛出NullPointerException。
在下面的示例中,我们使用空值创建一个字符串字面量。使用intern() 方法,我们尝试检索当前字符串对象的规范表示形式。
package com.tutorialspoint; public class Intern { public static void main(String[] args) { try { //create string literal String str = null; System.out.println("The given string is: " + str); //using the intern() method String str1 = str.intern(); System.out.println("The canonical representation of the current : " + str1); } catch(NullPointerException e) { e.printStackTrace(); System.out.println("Exception: " + e); } } }
输出
以下是上述程序的输出 -
The given string is: null java.lang.NullPointerException: Cannot invoke "String.intern()" because "str" is null at com.tutorialspoint.String.Intern.main(Intern.java:9) Exception: java.lang.NullPointerException: Cannot invoke "String.intern()" because "str" is null
检查创建当前字符串副本时的相等性示例
使用 intern() 方法,我们可以根据字符串对象的圆锥形来检查它们的相等性。
在此程序中,我们使用值“Hello”创建字符串类的对象。然后,使用intern() 方法,我们尝试检索字符串对象的规范表示形式,并使用equals() 方法进行比较。
package com.tutorialspoint; public class Intern { public static void main(String[] args) { // create an object of the string class String str = new String("Hello"); System.out.println("The given string is: " + str); // using intern() method String str2 = str.intern(); System.out.println("The canonical representation of the string object: " + str2); //using the conditional statement if (str.equals(str2)) { System.out.println("The string objects are equal based on their canonical forms"); } else { System.out.println("The string objects are not equal based on their canonical forms"); } } }
输出
上述程序产生以下输出 -
The given string is: Hello The canonical representation of the string object: Hello The string objects are equal based on their canonical forms
java_lang_string.htm
广告