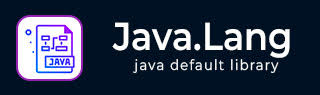
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包实用资源
- Java.lang - 实用资源
- Java.lang - 讨论
Java - String isEmpty() 方法
描述
Java String isEmpty() 方法用于检查当前字符串是否为空。该方法返回一个布尔值,当且仅当字符串为空时返回 true;否则返回 false。isEmpty() 方法不接受任何参数。
注意:如果字符串初始化为 null,isEmpty() 方法会抛出 NullPointerException。因为 Null 通常表示“此数据不可用”,它与空字符串不同,编译器无法处理它并抛出 NullPointerException。
语法
以下是 Java String isEmpty() 方法的语法:
public boolean isEmpty()
参数
该方法不接受任何参数。
返回值
如果 length() 为 0,则此方法返回 true,否则返回 false。
检查空字符串是否为空的示例
如果给定的字符串为空,isEmpty() 方法返回true。
在下面的程序中,我们创建一个具有空值的字符串字面量。然后,使用isEmpty() 方法,我们尝试检查当前字符串是否为空字符串。
package com.tutorialspoint; public class CheckEmpty { public static void main(String[] args) { //create a string literal String str = ""; System.out.println("The given string is an empty." + str); System.out.println("Length of the string is: " + str.length()); //using the isEmpty() method System.out.println("The current string is an empty string or not? " + str.isEmpty()); } }
输出
执行上述程序后,将产生以下结果:
The given string is an empty. Length of the string is: 0 The current string is an empty string or not? true
检查字符串是否为空的示例
如果给定的字符串非空,此方法返回false。
在下面的示例中,我们使用值"TutorialsPoint"实例化字符串类。使用isEmpty() 方法,我们尝试检查此字符串是否为空。
package com.tutorialspoint; public class CheckEmpty { public static void main(String[] args) { //instantiate the string class String str = new String("TutorialsPoint"); System.out.println("The given string is: " + str); System.out.println("Length of the string is: " + str.length()); //using the isEmpty() method System.out.println("The current string is an empty string or not? " + str.isEmpty()); } }
输出
以下是上述程序的输出:
The given string is: TutorialsPoint Length of the string is: 14 The current string is an empty string or not? false
检查字符串是否为空的示例
使用条件语句检查当前字符串是否为空。
在这个程序中,我们创建了一个值为"Hello"的字符串类对象。然后,使用isEmpty() 方法和条件语句,我们尝试检查当前字符串是否为空。
package com.tutorialspoint; public class CheckEmpty { public static void main(String[] args) { //create an object of the string class String str = new String("Hello"); System.out.println("The given string is: " + str); System.out.println("Length of the string is: " + str.length()); //using the isEmpty() method boolean bool = str.isEmpty(); if(bool) { System.out.println("The string is an empty string."); } else { System.out.println("The string is not an empty string"); } } }
输出
上述程序产生以下输出:
The given string is: Hello Length of the string is: 5 The string is not an empty string
检查字符串是否为空时出现空指针异常的示例
如果给定的字符串为null,此方法将抛出NullPointerException。
在下面的程序中,我们创建一个具有null值的字符串字面量。使用isEmpty() 方法,我们尝试检查给定的字符串是否为空。由于给定的字符串为 null,此方法会抛出异常。
public class CheckEmpty { public static void main(String[] args) { try { //create the string literal String str = null; System.out.println("The given string is: " + str); System.out.println("Length of the string is: " + str.length()); //using the isEmpty() method boolean bool = str.isEmpty(); if(bool) { System.out.println("The string is an empty string."); } else { System.out.println("The string is not an empty string"); } } catch(NullPointerException e) { System.out.println("Exception: " + e); } } }
输出
执行上述程序后,将产生以下结果:
The given string is: null Exception: java.lang.NullPointerException: Cannot invoke "String.length()" because "str" is null
java_lang_string.htm
广告