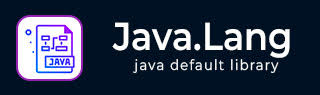
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包附加内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String lastIndexOf() 方法
Java String lastIndexOf() 方法用于检索指定字符在当前字符串中最后一次出现的索引位置。索引指的是字符串中字符的位置。
索引范围从0开始,到length() -1结束。第一个字符的值表示第 0 个索引,第二个字符的值表示第 1 个索引,以此类推。此方法接受不同的参数。
lastIndexOf() 方法有四个多态变体,具有不同的参数,例如 char、fromIndex 和字符串。以下是所有多态变体的语法。
语法
以下是Java String lastIndexOf() 方法的语法:
public int lastIndexOf(int ch) // first syntax public int lastIndexOf(int ch, int fromIndex)// second syntax public int lastIndexOf(String str)// third syntax public int lastIndexOf(String str, int fromIndex)// fourth syntax
参数
ch − 这是字符的 Unicode 码点值。
fromIndex − 这是索引的起始位置。
str − 这是字符串的值。
返回值
此方法返回在该字符串中指定字符最后一次出现的索引。// 第一个
此方法返回在该字符串中指定字符最后一次出现的索引,从指定的索引开始向后搜索。// 第二个
此方法返回在该字符串中指定子字符串最右边一次出现的索引。// 第三个
此方法返回在该字符串中指定子字符串最后一次出现的索引,从指定的索引开始向后搜索。// 第四个
示例
如果给定的字符值在当前字符串中没有出现,则 lastIndexOf() 方法返回-1。
在下面的程序中,我们使用值“Hello”实例化字符串类。使用lastIndexOf() 方法,我们尝试在当前字符串中检索字符'p'最后一次出现的索引值。
import java.lang.*; public class LastIndex { public static void main(String[] args) { //instantiate the string class String str = new String("Hello"); System.out.println("The given string is: " + str); //initialize the char value char ch = 'p'; System.out.println("The given char value is: " + ch); //using the lastIndexOf() method System.out.println("The position of the '" + ch + "' is: " + str.lastIndexOf(ch)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Hello The given char value is: p The position of the 'p' is: -1
示例
如果我们传递 char 和 fromIndex 作为参数给方法,此方法将返回在该字符串中指定字符最后一次出现的索引。
在下面的示例中,我们创建一个值为“HelloWorld”的字符串类对象。然后,使用lastIndexOf() 方法,我们尝试在指定fromIndex 5的字符串中检索字符“o”的位置。
import java.lang.*; public class LastIndex { public static void main(String[] args) { //create an object of the string class String str = new String("HelloWorld"); System.out.println("The given string is: " + str); //initialize the char value and fromIndex value char ch = 'o'; int fromIndex = 5; System.out.println("The given char and fromIndex values are: " + ch + " and " + fromIndex); //using the lastIndexOf() method System.out.println("The position of the '" + ch + "' is: " + str.lastIndexOf(ch, fromIndex)); } }
输出
以下是上述程序的输出:
The given string is: HelloWorld The given char and fromIndex values are: o and 5 The position of the 'o' is: 4
示例
如果我们传递字符串作为参数给方法,此方法将返回字符串在当前字符串中的位置。
在这个程序中,我们创建一个值为“TutorialsPoint”的字符串字面量。使用lastIndexOf() 方法,我们尝试在给定字符串值中检索指定字符串“als”最后一次出现的位置。
import java.lang.*; public class LastIndex { public static void main(String[] args) { //create string literal String str = "TutorialsPoint"; System.out.println("The given string is: " + str); //initialize argument string String str_argu = "als"; System.out.println("The argument string is: " + str_argu); //using the lastIndexOf() method System.out.println("The position of the '" + str_argu + "' is: " + str.lastIndexOf(str_argu)); } }
输出
上述程序产生以下输出:
The given string is: TutorialsPoint The argument string is: als The position of the 'als' is: 6
示例
如果我们传递字符串和 fromIndex 作为参数给方法,此方法将返回在该字符串中最后一次出现的索引。
在下面的程序中,我们使用值“Java Programming Language”实例化字符串类。使用lastIndexOf() 方法,我们尝试在指定fromIndex 10的当前字符串中检索字符串参数的位置。
import java.lang.*; public class LastIndex { public static void main(String[] args) { //instantiate the string class String str = new String("Java Programming Language"); System.out.println("The given string is: " + str); //initialize argument string and fromIndex value String str_argu = "Programming"; int fromIndex = 10; System.out.println("The given argument string and fromIndex values are: " + str_argu + " and " + fromIndex); //using the lastIndexOf() method System.out.println("The position of the '" + str_argu + "' is: " + str.lastIndexOf(str_argu, fromIndex)); } }
输出
执行上述程序后,将产生以下结果:
The given string is: Java Programming Language The given argument string and fromIndex values are: Programming and 10 The position of the 'Programming' is: 5