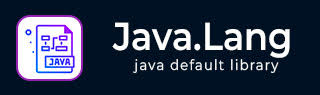
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String lastIndexOf() 方法
声明
以下是Java String lastIndexOf()方法的声明
public int lastIndexOf(int ch)
参数
ch − 这是字符(Unicode 代码点)的值。
返回值
此方法返回此对象表示的字符序列中字符最后一次出现的索引,如果字符不存在则返回 -1。
异常
无
Java - String lastIndexOf(int ch, int fromIndex) 方法
描述
Java String lastIndexOf(int ch, int fromIndex) 方法返回此字符串中指定字符最后一次出现的索引,从指定的索引开始向后搜索。
声明
以下是Java String lastIndexOf()方法的声明
public int lastIndexOf(int ch, int fromIndex)
参数
ch − 这是字符(Unicode 代码点)的值。
fromIndex − 这是开始搜索的索引。如果它大于或等于此字符串的长度,则其效果与等于此字符串长度减 1 相同:可以搜索整个字符串。如果它为负数,则其效果与等于 -1 相同:返回 -1。
返回值
此方法返回此对象表示的字符序列中小于或等于 fromIndex 的字符最后一次出现的索引,如果字符在该点之前不存在则返回 -1。
异常
无
Java - String lastIndexOf(String str) 方法
描述
java.lang.String.lastIndexOf(String str) 方法返回此字符串中指定子字符串最右边出现位置的索引。最右边的空字符串 "" 被认为出现在索引值 this.length() 处。
返回的索引是最大的值 k,使得this.startsWith(str, k) 为真。
声明
以下是Java String lastIndexOf()方法的声明
public int lastIndexOf(String str)
参数
str − 这是要搜索的子字符串。
返回值
如果字符串参数在此对象的子字符串中出现一次或多次,则返回最后一个此类子字符串的第一个字符的索引。如果它没有作为子字符串出现,则返回 -1。
异常
无
Java - String lastIndexOf(String str, int fromIndex) 方法
描述
Java String lastIndexOf(String str, int fromIndex) 方法返回此字符串中指定子字符串最后一次出现的索引,从指定的索引开始向后搜索。返回的整数是最大的值 k,使得 -
k >= Math.min(fromIndex, this.length()) && this.startsWith(str, k),如果不存在这样的 k 值,则返回 -1。
声明
以下是Java String lastIndexOf()方法的声明
public int lastIndexOf(String str, int fromIndex)
参数
str − 这是要搜索的子字符串。
fromIndex − 这是开始搜索的索引。
返回值
此方法返回此字符串中指定子字符串最后一次出现的索引。
异常
无
从字符串中获取字符的最后一个索引示例
以下示例演示了 Java String lastIndexOf() 方法的用法。我们创建了一个字符串字面量,其值为"This is tutorialspoint"。然后使用lastIndexOf()方法,我们检索e的索引。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "This is tutorialspoint"; // returns the index of last occurrence of character i System.out.println("last index of letter 'i' = " + str.lastIndexOf('i')); // returns -1 as character e is not in the string System.out.println("last index of letter 'e' = " + str.lastIndexOf('e')); } }
让我们编译并运行上述程序,这将产生以下结果 -
last index of letter 'i' = 19 last index of letter 'e' = -1
从字符串中获取字符的最后一个索引并带索引示例
以下示例演示了 Java String lastIndexOf() 方法的用法。我们创建了一个字符串字面量,其值为"This is tutorialspoint"。然后使用lastIndexOf()方法,我们检索e的索引。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "This is tutorialspoint"; /* returns positive value(last occurrence of character t) as character is located, which searches character t backward till index 14 */ System.out.println("last index of letter 't' = " + str.lastIndexOf('t', 14)); /* returns -1 as character is not located under the give index, which searches character s backward till index 2 */ System.out.println("last index of letter 's' = " + str.lastIndexOf('s', 2)); // returns -1 as character e is not in the string System.out.println("last index of letter 'e' = " + str.lastIndexOf('e', 5)); } }
让我们编译并运行上述程序,这将产生以下结果 -
last index of letter 't' = 10 last index of letter 's' = -1 last index of letter 'e' = -1
从字符串中获取字符串的最后一个索引示例
以下示例演示了 Java String lastIndexOf() 方法的用法。我们创建了一个字符串字面量,其值为"Collections of tutorials at tutorials point"。然后使用lastIndexOf()方法,我们检索tutorials的索引。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = "Collections of tutorials at tutorials point"; // returns index of first character of the last substring "tutorials" System.out.println("index = " + str1.lastIndexOf("tutorials")); // returns -1 as substring "admin" is not located System.out.println("index = " + str1.lastIndexOf("admin")); } }
让我们编译并运行上述程序,这将产生以下结果 -
index = 28 index = -1
从字符串中获取字符串的最后一个索引并带索引示例
以下示例演示了 Java String lastIndexOf() 方法的用法。我们创建了一个字符串字面量,其值为"Collections of tutorials at tutorials point"。然后使用lastIndexOf()方法,我们检索tutorials的索引。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = "Collections of tutorials at tutorials point"; /* search starts from index 17 and if located it returns the index of the first character of the last substring "tutorials" */ System.out.println("index = " + str1.lastIndexOf("tutorials", 17)); /* search starts from index 9 and returns -1 as substring "admin" is not located */ System.out.println("index = " + str1.lastIndexOf("admin", 9)); } }
让我们编译并运行上述程序,这将产生以下结果 -
index = 15 index = -1