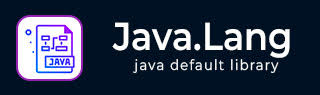
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包其他内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String matches() 方法
Java String matches() 方法用于检查当前字符串是否与给定的正则表达式匹配。正则表达式是一种模式,它指示字符串只能包含数字或字母字符。任何字符集或模式都可以用于正则表达式。
例如,我们经常需要构建代码来确定字符串是否为数字或是否包含字母;确定字符串是否包含特定数字四次;字符串是否仅由 A 到 Z 或 a 到 z 等字母组成等。Java 编程中有许多需要正则表达式的场景。
语法
以下是Java String matches()方法的语法:
public boolean matches(String regex)
参数
regex - 这是要与此字符串匹配的正则表达式。
返回值
当且仅当此字符串与给定的正则表达式匹配时,此方法返回 true。
匹配字符串示例
以下示例演示了 Java String matches() 方法的使用。这里我们创建了两个字符串 'str1' 和 'str2',其值分别为 "tutorials" 和 "learning"。然后,给定的字符串与提供的正则表达式进行匹配:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str1 = "tutorials", str2 = "learning"; boolean retval = str1.matches(str2); // method gets different values therefore it returns false System.out.println("Value returned = " + retval); retval = str2.matches("learning"); // method gets same values therefore it returns true System.out.println("Value returned = " + retval); retval = str1.matches("tuts"); // method gets different values therefore it returns false System.out.println("Value returned = " + retval); } }
输出
让我们编译并运行以上程序,这将产生以下结果
Value returned = false Value returned = true Value returned = false
使用 Pattern 进行字符串匹配示例
在下面的程序中,我们定义了一个用于数字匹配的正则表达式。当要匹配的字符串仅包含数字时,返回结果 "true";当包含字母和数字;数字和字符组合时,返回 "false",因为它不匹配正则表达式。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String RegexExpression = "^[0-9]+$"; System.out.println("The returned value is: " + "8785".matches(RegexExpression)); System.out.println("The returned value is: " + "@%*6575".matches(RegexExpression)); System.out.println("The returned value is: " + "675hjh".matches(RegexExpression)); } }
输出
让我们编译并运行上面的程序,输出将显示如下:
The returned value is: true The returned value is: false The returned value is: false
检查字符串是否仅包含字母字符示例
我们现在将创建一个程序来确定字符串是否仅包含字母字符。为此,应该提供包含“A 到 Z”、“a 到 z”和一个空格 (' ') 的正则表达式,因为字符串可能包含多个单词。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String RegexExpression = "[a-zA-Z ]+"; String s1 = "Tutorials Point is a reputed firm"; String s2 = "Tutori@ls && Po!nt is @ reputed f!rm"; String s3 = "Tutorials Point is a reputed firm 766868"; boolean Match1 = s1.matches("[a-zA-Z ]+"); boolean Match2 = s2.matches("[a-zA-Z ]+"); boolean Match3 = s3.matches("[a-zA-Z ]+"); System.out.println("Is the string having only alphabets: " + Match1); System.out.println("Is the string having only alphabets: " + Match2); System.out.println("Is the string having only alphabets: " + Match3); } }
输出
在执行以上程序时,输出如下所示
Is the string having only alphabets: true Is the string having only alphabets: false Is the string having only alphabets: false
获取无效模式的 PatternSyntaxException 示例
在下面给出的程序中,正则表达式定义不正确。由于语法错误,引发了一个异常:
public class StringDemo { public static void main(String[] args) { String s = "Coding"; String regexExpression = "[Cod...!!"; // printing the result System.out.println("The returned value is: " + s.matches(regexExpression)); } }
运行时异常
上面程序的输出如下所示
Exception in thread "main" java.util.regex.PatternSyntaxException: Unclosed character class near index 8 [Cod...!! ^ at java.base/java.util.regex.Pattern.error(Pattern.java:2038) at java.base/java.util.regex.Pattern.clazz(Pattern.java:2700) at java.base/java.util.regex.Pattern.sequence(Pattern.java:2149) at java.base/java.util.regex.Pattern.expr(Pattern.java:2079) at java.base/java.util.regex.Pattern.compile(Pattern.java:1793) at java.base/java.util.regex.Pattern.<init>(Pattern.java:1440) at java.base/java.util.regex.Pattern.compile(Pattern.java:1079) at java.base/java.util.regex.Pattern.matches(Pattern.java:1184) at java.base/java.lang.String.matches(String.java:2838) at StringDemo.main(StringDemo.java:6)
java_lang_string.htm
广告