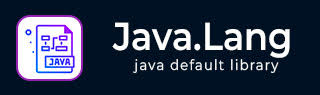
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包补充
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String offsetByCodePoints() 方法
Java String offsetByCodePoints() 方法返回字符串对象中从指定索引偏移 codePointOffset 个码点后的索引。
码点是 Unicode 系统中用于标识符号的数值。偏移量是正在使用的存储区的第一个索引。
文本范围内的不成对代理项将被视为各一个码点。
语法
以下是Java String offsetByCodePoints() 方法的语法:
public int offsetByCodePoints(int index, int codePointOffset)
参数
index − 这是要偏移的索引。
codePointOffset − 这是以码点为单位的偏移量。
返回值
此方法返回此字符串中的索引。
获取按码点偏移的示例
以下示例演示了 Java String offsetByCodePoints() 方法的用法。这里我们创建一个值为 'welcome to tutorialspoint' 的字符串。然后,我们尝试打印给定字符串中的索引。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "welcome to tutorialspoint"; System.out.println("string = " + str); // returns the index within this String int retval = str.offsetByCodePoints(2, 4); // prints the index System.out.println("index = " + retval); } }
输出
让我们编译并运行上面的程序,这将产生以下结果:
string = welcome to tutorialspoint index = 6
使用给定索引获取按码点偏移的示例
如果我们将索引值作为零传递给此方法,它将从开头返回字符串。
在下面的示例中,通过提供索引 0 来计算给定字符串序列 'Tutorials Point is a reputed firm!' 的长度:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // Initializing a CharSequence object. CharSequence c = "Tutorials Point is a reputed firm!"; System.out.println("The given string is: " + c); // Initializing the index and codePointOffset int Index = 0; int codePointOffset = c.length(); // Getting the index within the given character sequence. int res = Character.offsetByCodePoints(c, Index, codePointOffset); System.out.println("The resultant length of the string is: " + res); } }
输出
让我们编译并运行上面的程序,输出将显示如下:
The given string is: Tutorials Point is a reputed firm! The resultant length of the string is: 34
检查获取按码点偏移时出现的异常示例
如果索引为负数或大于给定序列的长度,则 offsetByCodePoints() 方法将抛出异常,如下例所示:
public class StringDemo { public static void main(String[] args) { // initializing the string buffer object StringBuffer x = new StringBuffer("Coding"); System.out.println("The string is: " + x); // getting the offsetByCodePoints on the index -6 and an offset of 8 int index = x.offsetByCodePoints(-6, 8); System.out.println("index of the offset -6, 8 is: " + index); } }
异常
运行上面的程序后,将获得如下输出:
The string is: Coding Exception in thread "main" java.lang.IndexOutOfBoundsException at java.base/java.lang.AbstractStringBuilder.offsetByCodePoints(AbstractStringBuilder.java:461) at java.base/java.lang.StringBuffer.offsetByCodePoints(StringBuffer.java:279) at StringDemo.main(StringDemo.java:7)
检查码点偏移量是正数还是负数的示例
以下示例显示,如果 codePointOffset 为正或负,并且子序列中的索引小于 codePointOffset 码点,则 offsetByCodePoints() 方法将抛出异常:
public class StringDemo { public static void main(String[] args) { // initializing the string buffer object StringBuffer x = new StringBuffer("Coding"); System.out.println("The string is: " + x); // getting the offsetByCodePoints on the index -6 and an offset of 8 int index = x.offsetByCodePoints(2, 5); System.out.println("index of the offset 2, 5 is: " + index); } }
异常
上面程序的输出如下:
The string is: Coding Exception in thread "main" java.lang.IndexOutOfBoundsException at java.base/java.lang.Character.offsetByCodePoints(Character.java:9342) at java.base/java.lang.AbstractStringBuilder.offsetByCodePoints(AbstractStringBuilder.java:463) at java.base/java.lang.StringBuffer.offsetByCodePoints(StringBuffer.java:279) at StringDemo.main(StringDemo.java:7)
java_lang_string.htm
广告