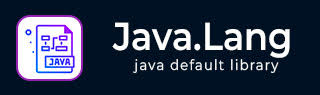
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String substring() 方法
Java String substring() 方法用于检索 String 对象的子字符串。子字符串从给定索引处的字符开始,一直持续到当前字符串的末尾。
由于 Java 中的 String 是不可变的,因此此方法始终返回一个新字符串,而不会更改之前的字符串。
此方法有两个多态变体,其语法如下所示;一个只允许您指定起始索引,另一个允许您同时指定起始索引和结束索引。
语法
以下是Java String substring()方法的语法
public String substring(int beginIndex) // first syntax or, public String substring(int beginIndex, int endIndex) // second syntax
注意 - 如果未指定 endIndex,则该方法将返回从 startIndex 开始的所有字符。
参数
beginIndex - 这是起始索引的值,包含在内。// 第一种语法
endIndex - 这是结束索引的值,不包含在内。// 第二种语法
返回值
此方法返回指定的子字符串。
示例:使用起始索引从字符串中获取子字符串
以下示例演示了通过查找字符串“This is tutorials point”的子字符串来使用 Java String substring() 方法。这里,只将起始索引作为参数传递给此方法:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "This is tutorials point"; String substr = ""; // prints the substring after index 7 substr = str.substring(7); System.out.println("substring = " + substr); // prints the substring after index 0 i.e whole string gets printed substr = str.substring(0); System.out.println("substring = " + substr); } }
输出
如果编译并运行上述程序,它将产生以下结果:
substring = tutorials point substring = This is tutorials point
示例:使用起始和结束索引从字符串中获取子字符串
以下是一个示例,演示了通过查找提供的字符串的子字符串在 Java 中使用 substring() 方法。这里,字符串的起始和结束位置都作为参数传递:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String str = "This is tutorials point"; String substr = ""; // prints the substring after index 7 till index 17 substr = str.substring(7, 17); System.out.println("substring = " + substr); // prints the substring after index 0 till index 7 substr = str.substring(0, 7); System.out.println("substring = " + substr); } }
输出
让我们编译并运行上面的程序,输出将显示如下:
substring = tutorials substring = This is
示例:从括号之间的字符串中获取子字符串
在这个示例中,我们尝试从字符串中检索括号内的子字符串。在这里,我们使用 indexOf() 方法查找左括号和右括号的索引。一旦我们获得这些索引,我们就使用 substring() 方法来获取此范围内的子字符串。
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String s = "Stabbed in the (back)"; // getting substring between '(' and ')' delimeter int begin = s.indexOf("("); int ter = s.indexOf(")"); String res = s.substring(begin + 1, ter); System.out.println("The substring is: " + res); } }
输出
执行上述程序后,输出如下:
The substring is: back
示例:从括号之间的字符串中获取子字符串
到目前为止,我们已经使用索引值来确定所需子字符串的边界。我们还可以打印两个给定字符串值之间存在的子字符串。以下是一个示例:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { String s = "Stabbed in the (back)"; // getting substring between '(' and ')' delimeter int begin = s.indexOf("(") + 1; int ter = s.indexOf(")"); String res = s.substring(begin, ter); System.out.println("The substring is: " + res); } }
输出
以下是上述程序的输出:
The substring is: back
示例:从字符串中删除第一个和最后一个字符
此程序中使用 substring() 方法从字符串中删除第一个和最后一个字符。
package com.tutorialspoint; public class StringDemo { public static void main(String args[]) { String s = new String("Programming"); System.out.print("Substring after eliminating the first character is: "); // excluding the first character since index(0) is excluded System.out.println(s.substring(1)); System.out.print("Substring after eliminating the last character is: "); // excluding the last character by providing index as -1 System.out.println(s.substring(0, s.length() - 1)); System.out.println( "Substring after eliminating both the first and the last character is: " + s.substring(1, s.length() - 1)); } }
输出
以下是上述代码的输出:
Substring after eliminating the first character is: rogramming Substring after eliminating the last character is: Programmin Substring after eliminating both the first and the last character is: rogrammin