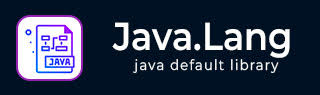
- Java.lang 包类
- Java.lang - 首页
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang 包额外内容
- Java.lang - 接口
- Java.lang - 错误
- Java.lang - 异常
- Java.lang 包有用资源
- Java.lang - 有用资源
- Java.lang - 讨论
Java - String toString() 方法
描述
此方法是从 Object 类重写而来。通常,toString() 方法返回当前对象的字符串表示形式。Java String toString() 方法用于检索字符串本身,并将结果表示为文本格式。因此,此处没有进行实际的转换。
Java 中的字符串是一组字符,它是 Java lang 类的对象。Java 中的字符串类用于创建和修改字符串。字符串在创建后其值不能被修改。无需在字符串对象上显式调用此方法,它只是返回当前字符串而无需进行任何更改。通常此方法隐式调用。
语法
以下是Java String toString() 方法的语法:
public String toString()
参数
此方法不接受任何参数。
返回值
此方法返回字符串本身。
获取字符串对象的字符串表示形式示例
在下面给出的示例中,创建了一个值为“Welcome to Tutorials Point”的 String 对象。然后,我们在创建的字符串上调用 toString() 方法:
package com.tutorialspoint; public class StringDemo { public static void main(String args[]) { String obj = new String("Welcome to Tutorials Point"); System.out.println("The string is: " + obj.toString()); } }
输出
如果编译并运行上面的程序,则输出将显示如下:
The string is: Welcome to Tutorials Point
获取整数的字符串表示形式示例
以下示例显示了 Java String toString() 方法的使用,用于将整数转换为其等效的字符串值:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // converts integer value in String representation int val1 = 325; String str1 = Integer.toString(val1); System.out.println("String representation of integer value = " + str1); } }
输出
如果编译并运行上述程序,它将产生以下结果:
String representation of integer value = 325
获取双精度数的字符串表示形式示例
下面是一个示例,使用 Java 中的 toString() 方法将双精度值转换为其等效字符串:
package com.tutorialspoint; public class StringDemo { public static void main(String[] args) { // converts double value in String representation double val2 = 876.23; String str2 = Double.toString(val2); System.out.println("String representation of double value = " + str2); } }
输出
执行上述程序后,输出如下所示:
String representation of double value = 876.23
获取对象的字符串表示形式示例
在下面的代码中,我们定义了一个名为 Vehicle 的类,并创建了它的两个对象 'v1' 和 'v2'。由于 Java 中的 toString() 方法在对象上工作,当打印 'v1' 和 'v2' 的值时,此方法被隐式调用。
class Vehicle { String brand; Integer modelNumber; Vehicle(String brand, Integer modelNumber) { // constructor to initialize variables of the class this.brand = brand; this.modelNumber = modelNumber; } } public class StringDemo { public static void main(String args[]) { Vehicle v1 = new Vehicle("Mahindra", 700); Vehicle v2 = new Vehicle("Maruti", 800); // The toString() method is called implicitly System.out.println("The value of object v1 is: " + v1); System.out.println("The value of object v2 is: " + v2); } }
输出
上面代码的输出分为两部分。第一部分是对象的类,第二部分是 toString() 方法返回的对象的十六进制代码,如下所示:
The value of object v1 is: Vehicle@2c7b84de The value of object v2 is: Vehicle@3fee733d
java_lang_string.htm
广告